lex和yacc实现计算器
时间: 2023-08-02 07:26:31 浏览: 177
Lex和Yacc是Unix下的常用工具,用来生成词法分析器和语法分析器。我们可以使用它们来实现简单的计算器。
首先,我们需要编写一个Lex文件,定义词法分析规则。这里我们需要定义数字和运算符的识别规则。
```lex
%{
#include <stdio.h>
#include "y.tab.h"
%}
%%
[0-9]+ { yylval = atoi(yytext); return NUMBER; }
[-+*/()] { return yytext[0]; }
[ \t\n] ; /* skip whitespace */
. { printf("Unknown token %s\n", yytext); }
%%
int yywrap() { return 1; }
```
上述代码中,%{ 和 %} 之间的代码是可选的C头文件。我们可以在其中包含需要的头文件。y.tab.h 是由Yacc生成的头文件,其中包含了语法分析器需要的一些定义。
接下来,我们需要编写一个Yacc文件,定义语法分析规则。这里我们定义了四则运算的语法规则,并在其中调用Lex生成的词法分析器。
```yacc
%{
#include <stdio.h>
#include <stdlib.h>
%}
%token NUMBER
%%
expression: NUMBER
| expression '+' expression
| expression '-' expression
| expression '*' expression
| expression '/' expression
| '(' expression ')'
;
%%
int main() {
yyparse();
return 0;
}
int yyerror(char* msg) {
printf("Error: %s\n", msg);
return 0;
}
```
上述代码中,%{ 和 %} 之间的代码是可选的C头文件。我们可以在其中包含需要的头文件。
接下来,我们可以使用以下命令生成词法分析器和语法分析器的代码:
```bash
lex calculator.l
yacc -d calculator.y
```
最后,我们需要编写一个main函数,调用语法分析器:
```c
#include <stdio.h>
#include "y.tab.h"
int main() {
yyparse();
return 0;
}
int yyerror(char* msg) {
printf("Error: %s\n", msg);
return 0;
}
```
现在我们可以编译并运行程序,输入表达式进行计算:
```bash
$ gcc lex.yy.c y.tab.c main.c -o calculator
$ ./calculator
1+2*3
```
输出结果为:
```bash
7
```
这就是使用Lex和Yacc实现计算器的基本步骤。
阅读全文
相关推荐
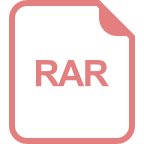
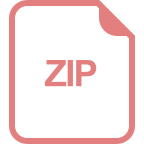



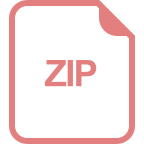
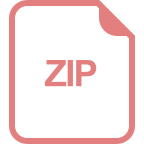
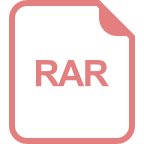
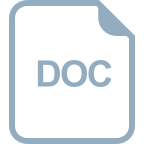
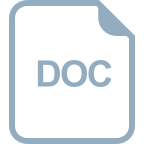
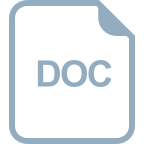



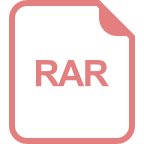
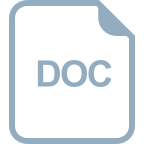
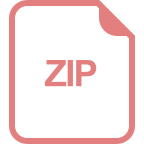