Linux字符设备驱动模板
时间: 2023-10-18 07:04:37 浏览: 162
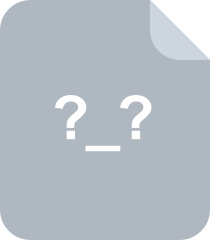
linux字符设备驱动模型
以下是一个简单的Linux字符设备驱动程序模板,供参考:
```c
#include <linux/module.h>
#include <linux/fs.h>
#include <linux/cdev.h>
#include <linux/device.h>
#include <linux/uaccess.h>
#define DEVICE_NAME "mychardev"
#define CLASS_NAME "mycharclass"
static int major_num = 0;
static struct class* mycharclass = NULL;
static struct device* mychardev = NULL;
static struct cdev mycdev;
static int mychardev_open(struct inode*, struct file*);
static int mychardev_release(struct inode*, struct file*);
static ssize_t mychardev_read(struct file*, char*, size_t, loff_t*);
static ssize_t mychardev_write(struct file*, const char*, size_t, loff_t*);
static struct file_operations mychardev_fops = {
.owner = THIS_MODULE,
.open = mychardev_open,
.release = mychardev_release,
.read = mychardev_read,
.write = mychardev_write,
};
static int __init mychardev_init(void) {
// allocate major number dynamically
if (alloc_chrdev_region(&major_num, 0, 1, DEVICE_NAME) < 0) {
return -1;
}
// create device class
if ((mycharclass = class_create(THIS_MODULE, CLASS_NAME)) == NULL) {
unregister_chrdev_region(major_num, 1);
return -1;
}
// create device
if ((mychardev = device_create(mycharclass, NULL, major_num, NULL, DEVICE_NAME)) == NULL) {
class_destroy(mycharclass);
unregister_chrdev_region(major_num, 1);
return -1;
}
// initialize cdev
cdev_init(&mycdev, &mychardev_fops);
mycdev.owner = THIS_MODULE;
// add cdev to kernel
if (cdev_add(&mycdev, major_num, 1) < 0) {
device_destroy(mycharclass, major_num);
class_destroy(mycharclass);
unregister_chrdev_region(major_num, 1);
return -1;
}
printk(KERN_INFO "mychardev: registered\n");
return 0;
}
static void __exit mychardev_exit(void) {
cdev_del(&mycdev);
device_destroy(mycharclass, major_num);
class_destroy(mycharclass);
unregister_chrdev_region(major_num, 1);
printk(KERN_INFO "mychardev: unregistered\n");
}
static int mychardev_open(struct inode* inodep, struct file* filep) {
printk(KERN_INFO "mychardev: opened\n");
return 0;
}
static int mychardev_release(struct inode* inodep, struct file* filep) {
printk(KERN_INFO "mychardev: closed\n");
return 0;
}
static ssize_t mychardev_read(struct file* filep, char* buffer, size_t len, loff_t* offset) {
printk(KERN_INFO "mychardev: read from device\n");
return 0;
}
static ssize_t mychardev_write(struct file* filep, const char* buffer, size_t len, loff_t* offset) {
printk(KERN_INFO "mychardev: wrote to device\n");
return len;
}
module_init(mychardev_init);
module_exit(mychardev_exit);
MODULE_LICENSE("GPL");
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("A simple character device driver");
```
需要注意的是,该模板仅提供了一个最基本的字符设备驱动程序框架,需要根据实际需求进行修改。此外,还需要在Makefile中添加相应的编译规则,将驱动程序编译成内核模块。
阅读全文
相关推荐

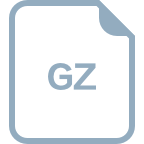
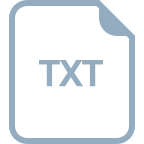
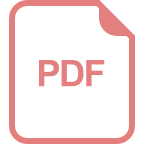
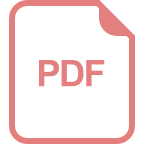
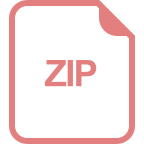
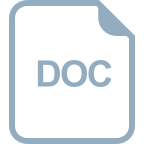
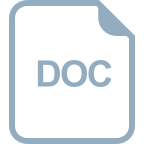
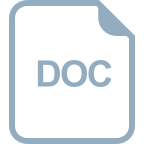
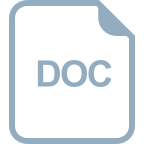
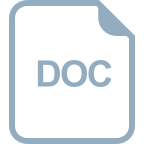

