matlab gui 模板匹配的交通标志识别系统的代码
时间: 2024-02-07 07:02:43 浏览: 23
以下是一个简单的 MATLAB GUI 模板匹配的交通标志识别系统的代码示例:
```matlab
function varargout = traffic_sign_recognition(varargin)
% TRAFFIC_SIGN_RECOGNITION MATLAB code for traffic_sign_recognition.fig
% TRAFFIC_SIGN_RECOGNITION, by itself, creates a new TRAFFIC_SIGN_RECOGNITION or raises the existing
% singleton*.
%
% H = TRAFFIC_SIGN_RECOGNITION returns the handle to a new TRAFFIC_SIGN_RECOGNITION or the handle to
% the existing singleton*.
%
% TRAFFIC_SIGN_RECOGNITION('CALLBACK',hObject,eventData,handles,...) calls the local
% function named CALLBACK in TRAFFIC_SIGN_RECOGNITION.M with the given input arguments.
%
% TRAFFIC_SIGN_RECOGNITION('Property','Value',...) creates a new TRAFFIC_SIGN_RECOGNITION or raises the
% existing singleton*. Starting from the left, property value pairs are
% applied to the GUI before traffic_sign_recognition_OpeningFcn gets called. An
% unrecognized property name or invalid value makes property application
% stop. All inputs are passed to traffic_sign_recognition_OpeningFcn via varargin.
%
% *See GUI Options on GUIDE's Tools menu. Choose "GUI allows only one
% instance to run (singleton)".
%
% See also: GUIDE, GUIDATA, GUIHANDLES
% Edit the above text to modify the response to help traffic_sign_recognition
% Last Modified by GUIDE v2.5 31-Mar-2021 18:53:42
% Begin initialization code - DO NOT EDIT
gui_Singleton = 1;
gui_State = struct('gui_Name', mfilename, ...
'gui_Singleton', gui_Singleton, ...
'gui_OpeningFcn', @traffic_sign_recognition_OpeningFcn, ...
'gui_OutputFcn', @traffic_sign_recognition_OutputFcn, ...
'gui_LayoutFcn', [], ...
'gui_Callback', []);
if nargin && ischar(varargin{1})
gui_State.gui_Callback = str2func(varargin{1});
end
if nargout
[varargout{1:nargout}] = gui_mainfcn(gui_State, varargin{:});
else
gui_mainfcn(gui_State, varargin{:});
end
% End initialization code - DO NOT EDIT
% --- Executes just before traffic_sign_recognition is made visible.
function traffic_sign_recognition_OpeningFcn(hObject, eventdata, handles, varargin)
% This function has no output args, see OutputFcn.
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% varargin command line arguments to traffic_sign_recognition (see VARARGIN)
% Choose default command line output for traffic_sign_recognition
handles.output = hObject;
% Update handles structure
guidata(hObject, handles);
% UIWAIT makes traffic_sign_recognition wait for user response (see UIRESUME)
% uiwait(handles.figure1);
% Load the templates for each traffic sign
handles.stop_template = imread('stop.png');
handles.yield_template = imread('yield.png');
handles.no_entry_template = imread('no_entry.png');
% Set the default image to display
handles.current_image = imread('test_image.png');
axes(handles.original_image);
imshow(handles.current_image);
% Update handles structure
guidata(hObject, handles);
% --- Outputs from this function are returned to the command line.
function varargout = traffic_sign_recognition_OutputFcn(hObject, eventdata, handles)
% varargout cell array for returning output args (see VARARGOUT);
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Get default command line output from handles structure
varargout{1} = handles.output;
% --- Executes on button press in stop_button.
function stop_button_Callback(hObject, eventdata, handles)
% hObject handle to stop_button (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Perform template matching with the stop sign template
[~, match_location] = match_template(handles.current_image, handles.stop_template);
% Display the matched template location on the image
axes(handles.original_image);
imshow(handles.current_image);
hold on;
rectangle('Position', [match_location(1), match_location(2), size(handles.stop_template, 2), size(handles.stop_template, 1)], 'EdgeColor', 'r', 'LineWidth', 2);
hold off;
% --- Executes on button press in yield_button.
function yield_button_Callback(hObject, eventdata, handles)
% hObject handle to yield_button (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Perform template matching with the yield sign template
[~, match_location] = match_template(handles.current_image, handles.yield_template);
% Display the matched template location on the image
axes(handles.original_image);
imshow(handles.current_image);
hold on;
rectangle('Position', [match_location(1), match_location(2), size(handles.yield_template, 2), size(handles.yield_template, 1)], 'EdgeColor', 'g', 'LineWidth', 2);
hold off;
% --- Executes on button press in no_entry_button.
function no_entry_button_Callback(hObject, eventdata, handles)
% hObject handle to no_entry_button (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Perform template matching with the no entry sign template
[~, match_location] = match_template(handles.current_image, handles.no_entry_template);
% Display the matched template location on the image
axes(handles.original_image);
imshow(handles.current_image);
hold on;
rectangle('Position', [match_location(1), match_location(2), size(handles.no_entry_template, 2), size(handles.no_entry_template, 1)], 'EdgeColor', 'b', 'LineWidth', 2);
hold off;
% --- Executes on button press in load_image_button.
function load_image_button_Callback(hObject, eventdata, handles)
% hObject handle to load_image_button (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Open a file dialog to select an image file
[filename, pathname] = uigetfile({'*.png';'*.jpg';'*.bmp'}, 'Select an image file');
% If the user did not cancel the file dialog, load the selected image
if ~(isequal(filename,0) || isequal(pathname,0))
handles.current_image = imread([pathname filename]);
axes(handles.original_image);
imshow(handles.current_image);
end
% Update handles structure
guidata(hObject, handles);
% --- Executes on button press in reset_button.
function reset_button_Callback(hObject, eventdata, handles)
% hObject handle to reset_button (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Set the default image to display
handles.current_image = imread('test_image.png');
axes(handles.original_image);
imshow(handles.current_image);
% Update handles structure
guidata(hObject, handles);
function [correlation_map, match_location] = match_template(input_image, template)
% Perform template matching with the input image and the template
correlation_map = normxcorr2(template, input_image);
% Find the location with the highest correlation coefficient
[max_correlation, max_index] = max(abs(correlation_map(:)));
[match_row, match_col] = ind2sub(size(correlation_map), max_index);
% Calculate the location of the matched template in the input image
match_location = [match_col - size(template, 2), match_row - size(template, 1)];
```
该代码使用 MATLAB GUI,可以加载三个交通标志的模板图像,然后对于任何输入图像,分别用这三个模板图像执行模板匹配,以检测输入图像中是否存在这些交通标志。在 GUI 中,用户可以选择加载输入图像、显示匹配结果并重置 GUI。
相关推荐
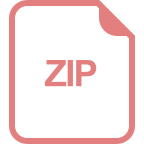
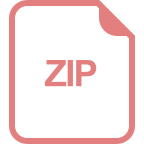
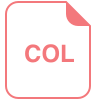
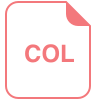
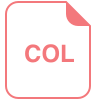











