如何使用Python中的pandas库来读取Excel文件中的两个特定工作表(子表),并将数据分别导入到Oracle数据库中?
时间: 2024-11-06 16:14:18 浏览: 76
在Python中,你可以使用pandas库来读取Excel文件,并通过`read_excel()`函数指定需要的工作表。然后,你需要连接到Oracle数据库,这通常可以借助于`cx_Oracle`库。以下是简单的步骤:
1. **安装所需库**:
首先,确保已经安装了pandas、openpyxl(用于读取Excel文件)、以及cx_Oracle库。如果还没有安装,可以使用pip安装:
```
pip install pandas openpyxl cx_Oracle
```
2. **读取Excel文件**:
使用pandas的`read_excel()`函数,传入Excel文件路径和需要的工作表名称列表:
```python
import pandas as pd
# 假设文件名为'myfile.xlsx',第一张表为'Sheet1',第二张表为'Sheet2'
df_sheet1 = pd.read_excel('myfile.xlsx', sheet_name='Sheet1')
df_sheet2 = pd.read_excel('myfile.xlsx', sheet_name='Sheet2')
```
3. **连接到Oracle数据库**:
使用`cx_Oracle.connect()`创建连接。这里假设用户名、密码和主机信息已知:
```python
import cx_Oracle
connection = cx_Oracle.connect(
user="your_username",
password="your_password",
dsn="your_database_dns"
)
```
4. **将数据导出到数据库**:
将每张表格的数据作为DataFrame操作,例如插入到不同的表中:
```python
# 假设你要将df_sheet1数据插入到表table1,df_sheet2数据插入到table2
for table, df in zip(['table1', 'table2'], [df_sheet1, df_sheet2]):
with connection.cursor() as cursor:
# 创建插入SQL语句
insert_query = f"INSERT INTO {table} ({df.columns.tolist()}) VALUES " + ", ".join([f"{tuple(row)}" for row in df.itertuples()])
# 执行插入
cursor.execute(insert_query)
```
5. **提交事务并关闭连接**:
最后别忘了关闭游标和连接:
```python
cursor.commit()
connection.close()
```
阅读全文
相关推荐
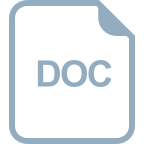
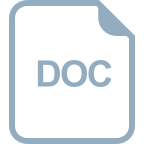
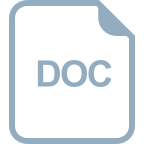
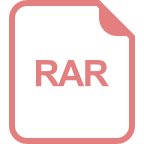
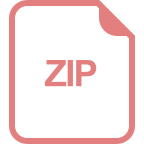
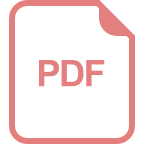
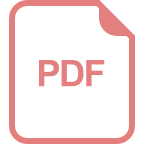
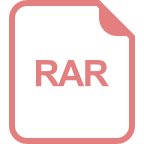
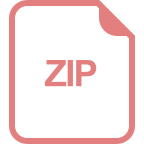
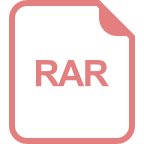
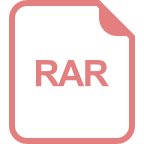
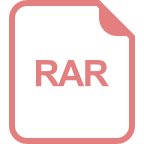
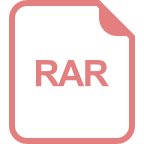
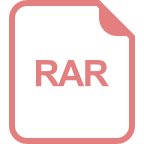
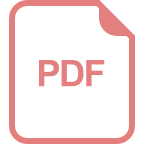
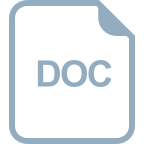
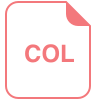
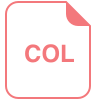
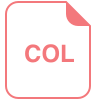