用C++写一个计算器的功能,使得计算器完成如下功能: 1. 四则运算,例如加减乘除,取余,开方,平方,立方等; 2. 除了整数的运算也可实现小数的各类运算; 3. 判断非法操作,例如判定1/0为非法操作; 4. 支持括号处理,; 5. 支持常见单目运算符的运算; 注意: 要理解表达式的逆波兰解析过程,推荐使用 QT框架实现图形化界面。
时间: 2024-03-05 13:53:13 浏览: 82
好的,我可以给你提供一个简单的示例代码,实现一个基本的计算器功能,包括四则运算、小数运算、非法操作判定、括号处理和单目运算符的运算。代码如下:
```cpp
#include <iostream>
#include <stack>
#include <queue>
#include <cmath>
#include <cstring>
using namespace std;
// 运算符优先级
int priority(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else if (op == '^') {
return 3;
} else {
return 0;
}
}
// 判断是否为数字
bool isDigit(char ch) {
return ch >= '0' && ch <= '9';
}
// 转换为数字
double toNumber(string str) {
return atof(str.c_str());
}
// 计算结果
double calculate(double num1, double num2, char op) {
switch (op) {
case '+':
return num1 + num2;
case '-':
return num1 - num2;
case '*':
return num1 * num2;
case '/':
if (num2 == 0) {
throw "Division by zero!";
}
return num1 / num2;
case '%':
if (num2 == 0) {
throw "Division by zero!";
}
return fmod(num1, num2);
case '^':
return pow(num1, num2);
default:
throw "Invalid operator!";
}
}
// 转换为逆波兰表达式
queue<string> toRPN(string expr) {
stack<char> opStack;
queue<string> rpnQueue;
string num = "";
for (int i = 0; i < expr.length(); i++) {
char ch = expr[i];
if (isDigit(ch) || ch == '.') {
num += ch;
} else {
if (!num.empty()) {
rpnQueue.push(num);
num = "";
}
if (ch == '(') {
opStack.push(ch);
} else if (ch == ')') {
while (!opStack.empty() && opStack.top() != '(') {
rpnQueue.push(string(1, opStack.top()));
opStack.pop();
}
if (opStack.empty()) {
throw "Mismatched parentheses!";
}
opStack.pop();
} else {
while (!opStack.empty() && opStack.top() != '(' && priority(opStack.top()) >= priority(ch)) {
rpnQueue.push(string(1, opStack.top()));
opStack.pop();
}
opStack.push(ch);
}
}
}
if (!num.empty()) {
rpnQueue.push(num);
}
while (!opStack.empty()) {
if (opStack.top() == '(') {
throw "Mismatched parentheses!";
}
rpnQueue.push(string(1, opStack.top()));
opStack.pop();
}
return rpnQueue;
}
// 计算逆波兰表达式
double calculateRPN(queue<string> rpnQueue) {
stack<double> numStack;
while (!rpnQueue.empty()) {
string token = rpnQueue.front();
rpnQueue.pop();
if (isDigit(token[0])) {
numStack.push(toNumber(token));
} else if (token.length() == 1 && !isDigit(token[0])) {
if (numStack.size() < 2) {
throw "Invalid expression!";
}
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(calculate(num1, num2, token[0]));
} else {
throw "Invalid expression!";
}
}
if (numStack.size() != 1) {
throw "Invalid expression!";
}
return numStack.top();
}
int main() {
string expr;
cout << "Enter an expression: ";
getline(cin, expr);
try {
queue<string> rpnQueue = toRPN(expr);
double result = calculateRPN(rpnQueue);
cout << "Result: " << result << endl;
} catch (const char* msg) {
cerr << "Error: " << msg << endl;
}
return 0;
}
```
该代码使用了栈和队列来实现逆波兰表达式的转换和计算,其中:
- `priority` 函数用于获取运算符的优先级;
- `isDigit` 函数用于判断字符是否为数字;
- `toNumber` 函数用于将字符串转换为浮点数;
- `calculate` 函数用于计算两个数字的运算结果;
- `toRPN` 函数用于将中缀表达式转换为逆波兰表达式;
- `calculateRPN` 函数用于计算逆波兰表达式的值;
- `main` 函数用于读入用户输入的表达式并调用上述函数进行计算。
该代码还包括异常处理,可以检测非法操作、除以零以及括号不匹配等错误,并输出错误信息。
阅读全文
相关推荐
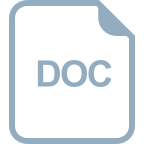
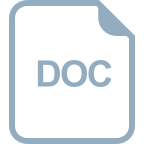
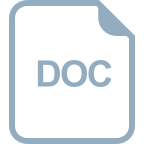
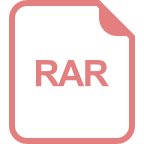
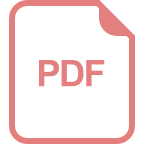
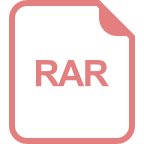
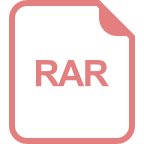
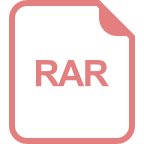
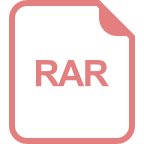
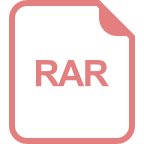
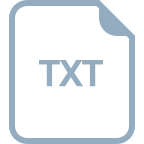
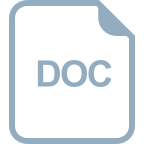
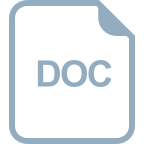




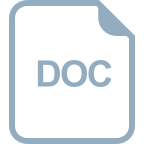
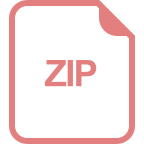