NumberUtil工具类
时间: 2023-10-05 09:10:57 浏览: 48
NumberUtil 是一个常用的工具类,用于对数字进行格式化、转换等操作。该类通常包含以下方法:
1. formatMoney(double money, int decimalDigits):将一个 double 类型的金额格式化为字符串,并保留指定位数的小数。
2. formatNumber(double number, int decimalDigits):将一个 double 类型的数字格式化为字符串,并保留指定位数的小数。
3. formatPercentage(double percentage, int decimalDigits):将一个 double 类型的百分数格式化为字符串,并保留指定位数的小数。
4. round(double number, int decimalDigits):将一个 double 类型的数字四舍五入为指定位数的小数。
5. parseDouble(String str):将一个字符串转换为 double 类型的数字。
6. parseInt(String str):将一个字符串转换为 int 类型的数字。
7. isNumber(String str):判断一个字符串是否为数字。
这些方法可以方便地对数字进行格式化、转换和判断,提高代码的可读性和可维护性。
相关问题
NumberUtil工具类编写
NumberUtil是一个常用的工具类,可以帮助我们在Java编程中快速处理数字类型的数据。下面是一个简单的NumberUtil工具类的实现:
```java
public class NumberUtil {
// 判断字符串是否是数字
public static boolean isNumber(String str) {
if (str == null || str.length() == 0) {
return false;
}
for (int i = 0; i < str.length(); i++) {
if (!Character.isDigit(str.charAt(i))) {
return false;
}
}
return true;
}
// 将字符串转换为int类型
public static int parseInt(String str) {
if (isNumber(str)) {
return Integer.parseInt(str);
} else {
throw new NumberFormatException("Not a number: " + str);
}
}
// 将字符串转换为double类型
public static double parseDouble(String str) {
if (isNumber(str)) {
return Double.parseDouble(str);
} else {
throw new NumberFormatException("Not a number: " + str);
}
}
// 将int类型转换为字符串
public static String toString(int num) {
return Integer.toString(num);
}
// 将double类型转换为字符串
public static String toString(double num) {
return Double.toString(num);
}
}
```
这个NumberUtil工具类包含了几个常用的方法:isNumber用于判断一个字符串是否是数字;parseInt和parseDouble用于将字符串转换为int和double类型;toString用于将int和double类型转换为字符串类型。
使用这个工具类,我们可以更加方便地处理数字类型的数据。例如,我们可以使用NumberUtil.parseInt方法将一个字符串转换为int类型:
```java
String str = "123";
int num = NumberUtil.parseInt(str);
System.out.println(num); // 输出:123
```
当然,如果字符串不是数字,NumberUtil.parseInt方法也会抛出NumberFormatException异常。因此,我们需要在使用这个方法时进行异常处理,以避免程序出错。
总之,NumberUtil工具类提供了一些方便的方法,可以帮助我们更加方便地处理数字类型的数据。在编写Java程序时,我们可以根据自己的需求来实现更多的方法,以提高代码的复用性和可维护性。
NumberUtil工具类编码
以下是一个简单的 NumberUtil 工具类的编码示例,包含了常用的数字处理方法:
```java
public class NumberUtil {
/**
* 判断一个字符串是否是数字
*
* @param str 字符串
* @return 如果是数字返回 true,否则返回 false
*/
public static boolean isNumeric(String str) {
if (str == null || str.length() == 0) {
return false;
}
for (int i = 0; i < str.length(); i++) {
if (!Character.isDigit(str.charAt(i))) {
return false;
}
}
return true;
}
/**
* 将字符串转换为整数,如果字符串不是数字,则返回 0
*
* @param str 字符串
* @return 整数
*/
public static int parseInt(String str) {
if (str == null || str.length() == 0) {
return 0;
}
int result = 0;
try {
result = Integer.parseInt(str);
} catch (NumberFormatException e) {
e.printStackTrace();
}
return result;
}
/**
* 将字符串转换为浮点数,如果字符串不是数字,则返回 0.0
*
* @param str 字符串
* @return 浮点数
*/
public static double parseDouble(String str) {
if (str == null || str.length() == 0) {
return 0.0;
}
double result = 0.0;
try {
result = Double.parseDouble(str);
} catch (NumberFormatException e) {
e.printStackTrace();
}
return result;
}
/**
* 将一个整数转换为指定长度的字符串,如果长度不足则在前面补 0
*
* @param num 整数
* @param length 长度
* @return 指定长度的字符串
*/
public static String padLeft(int num, int length) {
return String.format("%0" + length + "d", num);
}
}
```
这个工具类包含了以下方法:
- `isNumeric`:判断一个字符串是否是数字。
- `parseInt`:将字符串转换为整数。
- `parseDouble`:将字符串转换为浮点数。
- `padLeft`:将一个整数转换为指定长度的字符串,如果长度不足则在前面补 0。
这些方法可以帮助我们在处理数字时更加方便地进行操作。
相关推荐
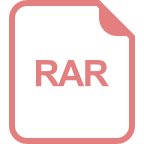
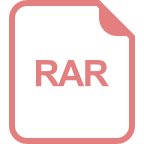








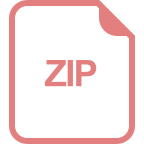
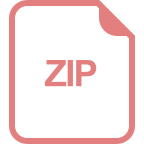
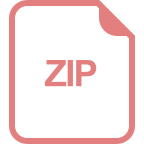
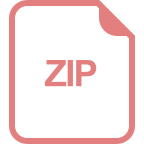
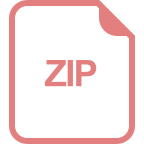