html js css带指针抽奖大转盘
时间: 2023-09-06 13:09:16 浏览: 52
以下是一个简单的 HTML、CSS、JavaScript 实现带指针的抽奖大转盘的示例代码:
HTML 代码:
```html
<div class="container">
<div class="wheel">
<div class="section"><span>1</span></div>
<div class="section"><span>2</span></div>
<div class="section"><span>3</span></div>
<div class="section"><span>4</span></div>
<div class="section"><span>5</span></div>
<div class="section"><span>6</span></div>
<div class="section"><span>7</span></div>
<div class="section"><span>8</span></div>
</div>
<div class="pointer"></div>
<button class="btn-spin">SPIN</button>
</div>
```
CSS 代码:
```css
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.wheel {
position: relative;
width: 300px;
height: 300px;
border-radius: 50%;
background: #fff;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.2);
overflow: hidden;
}
.section {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
border-radius: 50%;
transform-origin: 50% 100%;
}
.section:nth-child(1) {
transform: rotate(0deg) skewY(30deg);
background: #e74c3c;
}
.section:nth-child(2) {
transform: rotate(45deg) skewY(30deg);
background: #2ecc71;
}
.section:nth-child(3) {
transform: rotate(90deg) skewY(30deg);
background: #3498db;
}
.section:nth-child(4) {
transform: rotate(135deg) skewY(30deg);
background: #f1c40f;
}
.section:nth-child(5) {
transform: rotate(180deg) skewY(30deg);
background: #9b59b6;
}
.section:nth-child(6) {
transform: rotate(225deg) skewY(30deg);
background: #1abc9c;
}
.section:nth-child(7) {
transform: rotate(270deg) skewY(30deg);
background: #e67e22;
}
.section:nth-child(8) {
transform: rotate(315deg) skewY(30deg);
background: #f39c12;
}
.section span {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%) rotate(-30deg);
font-size: 24px;
font-weight: bold;
color: #fff;
}
.pointer {
position: absolute;
top: 50%;
left: 50%;
width: 2px;
height: 100px;
background: #e74c3c;
transform-origin: bottom;
transform: translate(-50%, -100%);
border-radius: 2px;
}
.btn-spin {
margin-top: 20px;
padding: 10px 20px;
font-size: 20px;
font-weight: bold;
color: #fff;
background: #e74c3c;
border: none;
border-radius: 50px;
cursor: pointer;
outline: none;
}
```
JavaScript 代码:
```javascript
const wheel = document.querySelector('.wheel');
const pointer = document.querySelector('.pointer');
const btnSpin = document.querySelector('.btn-spin');
let deg = 0;
let speed = 0;
let prize = '';
let running = false;
const sections = [
{ id: 1, deg: 0 },
{ id: 2, deg: 45 },
{ id: 3, deg: 90 },
{ id: 4, deg: 135 },
{ id: 5, deg: 180 },
{ id: 6, deg: 225 },
{ id: 7, deg: 270 },
{ id: 8, deg: 315 },
];
function rotateWheel() {
deg += speed;
wheel.style.transform = `rotate(${deg}deg)`;
const currentSection = sections.find(
(section) => deg >= section.deg - 1 && deg < section.deg + 1
);
pointer.style.transform = `rotate(${currentSection.deg + 30}deg)`;
if (running) {
requestAnimationFrame(rotateWheel);
} else {
setTimeout(() => {
alert(`Congratulations! You won ${prize}!`);
btnSpin.disabled = false;
}, 500);
}
}
btnSpin.addEventListener('click', () => {
if (!running) {
btnSpin.disabled = true;
prize = '';
speed = Math.random() * 30 + 10;
const selectedSection = sections[Math.floor(Math.random() * sections.length)];
prize = `prize ${selectedSection.id}`;
running = true;
requestAnimationFrame(rotateWheel);
}
});
```
在这个代码中,我们使用了 CSS 中的 `transform` 属性来实现大转盘的旋转和扇形区块的倾斜。JavaScript 实现了指针的旋转和大转盘的自动旋转功能,并且在抽奖结束时弹出中奖提示框。
相关推荐
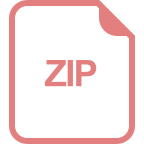
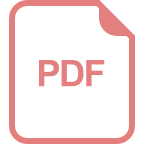












