使用C语言写出简单页面置换算法最久未被使用算法模拟程序
时间: 2024-11-21 09:20:45 浏览: 49
在C语言中,编写一个简单的页面置换算法(如最久未使用(LRU)算法)的模拟程序可以分为以下几个步骤:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// 定义页面结构
typedef struct Page {
int address; // 页面地址
bool is_recent; // 最近是否被访问标志
} Page;
// LRU缓存队列
typedef struct LRUCache {
Page* pages;
size_t capacity; // 缓存容量
size_t count; // 当前已使用的页面数
Page head; // 队首节点
Page tail; // 队尾节点
} LRUCache;
void initialize_LRUCache(LRUCache* cache, size_t capacity) {
cache->pages = (Page*)malloc(capacity * sizeof(Page));
cache->capacity = capacity;
cache->count = 0;
cache->head.address = -1;
cache->tail.address = -1;
}
// 插入页面到LRU队列
void insert_page(LRUCache* cache, int address) {
if (cache->count == cache->capacity) { // 如果满
Page* victim = &cache->pages[cache->tail.address]; // 将最久未使用的页面淘汰
victim->is_recent = false;
cache->tail = cache->tail.previous; // 更新队尾
free(victim);
cache->count--;
}
Page* new_page = &cache->pages[cache->count];
new_page->address = address;
new_page->is_recent = true;
cache->count++;
if (cache->count == 1 || cache->head.address != -1) { // 新页成为队首
cache->head.next = new_page;
new_page->previous = &cache->head;
cache->head = new_page;
} else { // 新页加到最后
cache->tail.next = new_page;
new_page->previous = &cache->tail;
cache->tail = new_page;
}
}
// 模拟访问并检查替换策略
void simulate_access(LRUCache* cache, int* accesses) {
for (size_t i = 0; i < 5; ++i) { // 假设有五个访问请求
printf("Accessing page %d\n", accesses[i]);
if (cache->head.address == accesses[i]) { // 如果是最新的页面
cache->head.is_recent = true;
} else {
insert_page(cache, accesses[i]); // 否则,插入并可能替换最久未使用的页面
}
}
}
int main() {
LRUCache cache;
initialize_LRUCache(&cache, 3); // 初始化一个大小为3的缓存
int accesses[] = {1, 2, 3, 4, 5}; // 访问顺序
simulate_access(&cache, accesses);
return 0;
}
```
在这个示例中,我们创建了一个简单的LRU缓存结构,并实现了初始化、插入页面和模拟访问功能。注意,这个程序仅用于演示基本的思路,并非高效的C++版本,因为C语言的标准库并不提供复杂的数据结构操作。
阅读全文
相关推荐
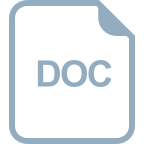
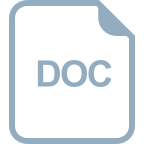
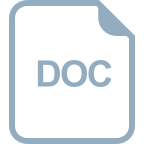




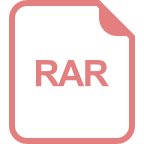
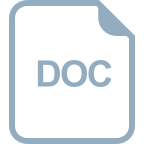


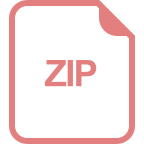
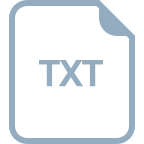
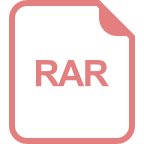
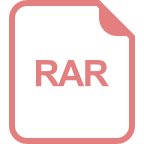
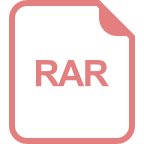
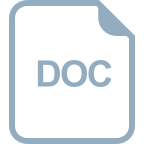


