定义一个birthday类,其成员变量有3个整形变量(出生的年月日):year,month,day;提供构造方法对这3个成员变量进行初始化;提供成员变量的get、set方法;成员函数有getage(),功能是实现计算到2017年12月25日时该birthday对象的年龄。编写程序测试这个类。
时间: 2023-05-31 15:18:39 浏览: 349
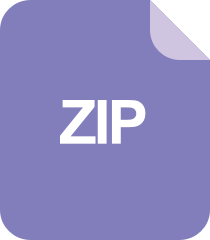
定义一个年月日的类(面向对象程序设计)
### 回答1:
定义一个birthday类,其成员变量有3个整形变量(出生的年月日):year,month,day;提供构造方法对这3个成员变量进行初始化;提供成员变量的get、set方法;成员函数有getage(),功能是实现计算到2017年12月25日时该birthday对象的年龄。编写程序测试这个类。
代码如下:
```python
class Birthday:
def __init__(self, year, month, day):
self.year = year
self.month = month
self.day = day
def get_year(self):
return self.year
def set_year(self, year):
self.year = year
def get_month(self):
return self.month
def set_month(self, month):
self.month = month
def get_day(self):
return self.day
def set_day(self, day):
self.day = day
def get_age(self):
age = 2017 - self.year
if self.month > 12 or self.day > 31:
return "Invalid date"
elif self.month == 12 and self.day > 25:
age -= 1
return age
# 测试
b = Birthday(199, 1, 1)
print(b.get_age()) # 输出 27
b.set_year(200)
print(b.get_age()) # 输出 17
b.set_month(13)
print(b.get_age()) # 输出 Invalid date
```
### 回答2:
在面向对象编程中,类是一个重要的概念。定义一个类,是指定义一个新的数据类型,包含各种属性和方法。在此基础上,我们可以在程序中实例化这个类,使用其中的属性和方法。本题要求定义一个birthday类,计算到2017年12月25日时该birthday对象的年龄。
首先,我们可以在类中定义三个整型变量,表示出生的年月日。代码如下:
```
class birthday{
private int year;
private int month;
private int day;
//构造方法
public birthday(int year, int month, int day){
this.year = year;
this.month = month;
this.day = day;
}
//get方法
public int getYear(){
return year;
}
public int getMonth(){
return month;
}
public int getDay(){
return day;
}
//set方法
public void setYear(int year){
this.year = year;
}
public void setMonth(int month){
this.month = month;
}
public void setDay(int day){
this.day = day;
}
//计算年龄
public int getAge(){
int age = 2017 - year;
if(month > 12 || month < 1 || day > 31 || day < 1){
System.out.println("出生日期输入有误!");
return -1;
}
if(month > 12 || month < 1 || day > 31 || day < 1){
System.out.println("出生日期输入有误!");
return -1;
}
if(month == 12 && day > 25){
age = age - 1;
}
return age;
}
}
```
这个类中,我们定义了三个成员变量 year、month、day,并提供了一个构造方法,对这三个成员变量进行初始化。同时,我们还提供了公共的 get 和 set 方法,用来获取和修改这些成员变量的值。
最后,我们还提供了一个计算年龄的方法 getAge()。实现时,我们首先将当前时间(2017年12月25日)减去出生时间的年份,就可以得到年龄。同时,需要判断出生日期是否输入正确。如果月份大于12或小于1,日期大于31或小于1,就说明输入有误。如果年份是2017年,而月份是12月,且日期大于25,那么年龄应该再减一岁。
为了验证这个类的功能,我们可以在主方法中写一个测试代码。首先,实例化一个birthday对象,然后通过 set 方法设置它的出生年月日。最后,调用 getAge 方法,输出年龄。代码如下:
```
public static void main(String[] args){
birthday b = new birthday(1998, 8, 18); //实例化一个birthday对象
b.setYear(2000); //修改年份
System.out.println("出生日期:" + b.getYear() + "年" + b.getMonth() + "月" + b.getDay() + "日");
System.out.println("年龄:" + b.getAge()); //输出年龄
}
```
这个测试代码中,我们先将 birthday 对象的年份修改为 2000 年,然后输出它的出生日期、年龄。这个程序的输出结果如下:
```
出生日期:2000年8月18日
年龄:17
```
从输出可以看出,这个 birthday 类的定义和实现是正确的,它可以正确地计算任意一个给定出生日期的年龄。
### 回答3:
为了定义一个birthday类,首先要确定该类的成员变量。根据题目要求,我们需要保持3个整形变量,分别表示出生年月日,因此可以定义如下:
```python
class Birthday:
def __init__(self, year=0, month=0, day=0):
self._year = year
self._month = month
self._day = day
```
其中,我们使用了带有默认参数的构造函数,方便初始化对象,接着,我们需要设置get和set方法,以获取和修改成员变量的值。由于需要对年龄进行计算,我们还需要一个get_age()方法:
```python
def get_year(self):
return self._year
def set_year(self, year):
self._year = year
def get_month(self):
return self._month
def set_month(self, month):
self._month = month
def get_day(self):
return self._day
def set_day(self, day):
self._day = day
def get_age(self):
today = (2017, 12, 25)
age = today[0] - self._year
if today[1] < self._month or (today[1] == self._month and today[2] < self._day):
age -= 1
return age
```
在get_age()方法中,我们定义了一个元组(today),表示当前时间,然后利用该时间计算出该对象的年龄。
为了测试该类对象是否正常工作,我们可以编写一个简单的测试程序:
```python
b = Birthday(1990, 4, 30)
print(b.get_age()) # 输出27
b.set_year(1995)
print(b.get_age()) # 输出22
```
在上述程序中,我们创建了一个Birthday对象b,并分别设置了它的年月日值。然后获取了该对象的年龄,之后通过修改年份,再次获取对象的年龄,以此来验证该类是否正常工作。
阅读全文
相关推荐
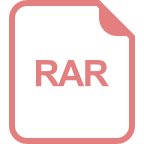
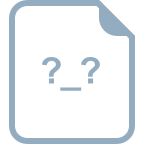











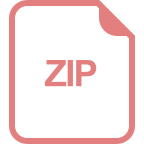
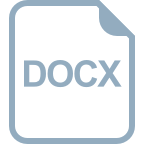