用c语言完整地编写⼀个链表的增删查API
时间: 2023-11-30 13:02:39 浏览: 55
好的,以下是使用 C 语言编写的链表增删查 API 的完整程序:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 数据域
struct Node *next; // 指针域
} Node;
// 定义链表头结点
Node *head = NULL;
// 在链表尾部插入节点
void insertNode(int data) {
Node *newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
newNode->data = data;
newNode->next = NULL;
if (head == NULL) { // 如果链表为空,则新节点为头节点
head = newNode;
return;
}
Node *temp = head;
while (temp->next != NULL) { // 遍历链表找到尾节点
temp = temp->next;
}
temp->next = newNode; // 将新节点插入到尾节点的后面
}
// 删除链表中指定的节点
void deleteNode(int data) {
if (head == NULL) { // 如果链表为空,则无需删除
return;
}
if (head->data == data) { // 如果头节点为要删除的节点
Node *temp = head;
head = head->next; // 将头指针指向下一个节点
free(temp); // 释放原头节点
return;
}
Node *prev = head; // 记录上一个节点
Node *temp = head->next; // 记录当前节点
while (temp != NULL) {
if (temp->data == data) { // 如果找到要删除的节点
prev->next = temp->next; // 将上一个节点的指针指向下一个节点
free(temp); // 释放当前节点
return;
}
prev = temp; // 上一个节点指针指向当前节点
temp = temp->next; // 当前节点指针指向下一个节点
}
}
// 查找链表中指定的节点
Node* searchNode(int data) {
Node *temp = head;
while (temp != NULL) {
if (temp->data == data) { // 如果找到要查找的节点
return temp; // 返回该节点指针
}
temp = temp->next; // 当前节点指针指向下一个节点
}
return NULL; // 如果未找到,则返回空指针
}
// 输出链表中的所有节点
void printList() {
if (head == NULL) { // 如果链表为空,则无需输出
printf("链表为空!\n");
return;
}
Node *temp = head;
while (temp != NULL) { // 遍历链表输出每个节点的数据
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
// 主函数
int main() {
insertNode(1);
insertNode(2);
insertNode(3);
insertNode(4);
printList(); // 输出链表中的所有节点
deleteNode(2);
deleteNode(4);
printList(); // 输出链表中的所有节点
Node *node = searchNode(3);
if (node != NULL) {
printf("查找到节点:%d\n", node->data);
} else {
printf("未找到节点!\n");
}
return 0;
}
```
在程序中,我们先定义了链表节点结构体 `Node`,其中包含两个成员变量:`data` 表示节点中存储的数据,`next` 表示指向下一个节点的指针。然后定义了链表头结点 `head`,初始化为 `NULL`。
接着,定义了四个链表操作函数:
- `insertNode`:在链表尾部插入节点。
- `deleteNode`:删除链表中指定的节点。
- `searchNode`:查找链表中指定的节点。
- `printList`:输出链表中的所有节点。
最后在 `main` 函数中调用这些函数,实现链表的增删查操作。
阅读全文
相关推荐

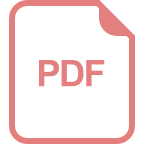
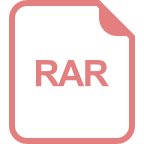
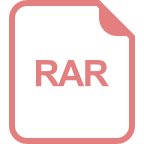
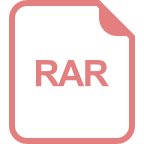
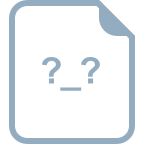
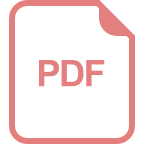
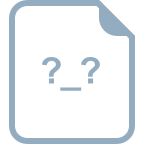
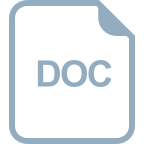
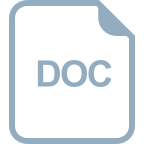