用C++ 编写一个学生和教师的数据输入和显示程序。学生数据有编号、姓名、性别、年龄、系别和成绩,教师数据有编号、姓名、性别、年龄、职称和部门。要求将编号、姓名、性别、年龄的输入和显示设计成一个类Person,并作为学生类Student和教师类Teacher的基类。
时间: 2023-12-22 08:20:06 浏览: 66
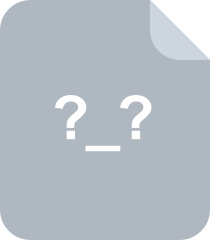
编写一个学生和教师数据输入和显示程序
以下是一个简单的实现,包括Person类和Student、Teacher类的定义和实现。其中,Person类包含编号、姓名、性别和年龄等基本信息,Student类和Teacher类分别继承Person类,增加了学生和教师特有的信息和成员函数。
```c++
#include <iostream>
#include <string>
using namespace std;
// 基类Person
class Person {
protected:
string id; // 编号
string name; // 姓名
char gender; // 性别
int age; // 年龄
public:
Person(string _id="", string _name="", char _gender='M', int _age=0)
: id(_id), name(_name), gender(_gender), age(_age) {}
virtual void input() {
cout << "请输入编号:"; cin >> id;
cout << "请输入姓名:"; cin >> name;
cout << "请输入性别(M/F):"; cin >> gender;
cout << "请输入年龄:"; cin >> age;
}
virtual void display() {
cout << "编号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "年龄:" << age << endl;
}
};
// 学生类Student,继承自Person
class Student : public Person {
private:
string major; // 专业
double score; // 成绩
public:
Student(string _id="", string _name="", char _gender='M', int _age=0, string _major="", double _score=0.0)
: Person(_id, _name, _gender, _age), major(_major), score(_score) {}
void input() {
Person::input();
cout << "请输入专业:"; cin >> major;
cout << "请输入成绩:"; cin >> score;
}
void display() {
Person::display();
cout << "专业:" << major << endl;
cout << "成绩:" << score << endl;
}
};
// 教师类Teacher,继承自Person
class Teacher : public Person {
private:
string title; // 职称
string department; // 部门
public:
Teacher(string _id="", string _name="", char _gender='M', int _age=0, string _title="", string _department="")
: Person(_id, _name, _gender, _age), title(_title), department(_department) {}
void input() {
Person::input();
cout << "请输入职称:"; cin >> title;
cout << "请输入部门:"; cin >> department;
}
void display() {
Person::display();
cout << "职称:" << title << endl;
cout << "部门:" << department << endl;
}
};
int main() {
Person *p;
Student s;
Teacher t;
// 输入并显示学生信息
p = &s;
p->input();
p->display();
// 输入并显示教师信息
p = &t;
p->input();
p->display();
return 0;
}
```
阅读全文
相关推荐
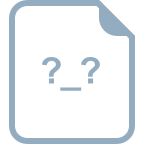



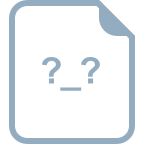
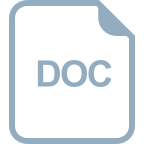
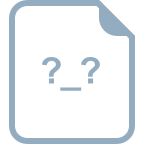
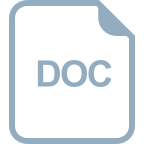
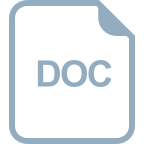
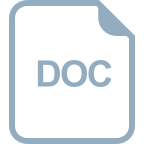
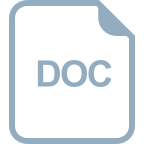
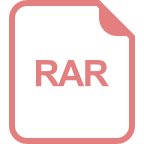
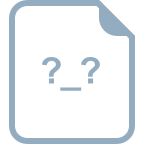
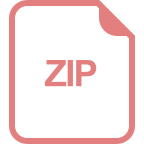