SpringBoot 使用 Caffeine 教程
时间: 2024-01-24 13:02:52 浏览: 117
好的,下面是 SpringBoot 使用 Caffeine 的教程:
1. 引入依赖
在 pom.xml 文件中引入 Caffeine 依赖:
```xml
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
<version>2.9.0</version>
</dependency>
```
2. 配置 Caffeine 缓存
在 SpringBoot 的配置文件中添加以下配置:
```yaml
spring.cache.type=caffeine
spring.cache.caffeine.spec=maximumSize=100,expireAfterAccess=5m
```
这里使用了 maximumSize 和 expireAfterAccess 两个参数,maximumSize 表示缓存的最大容量为 100,expireAfterAccess 表示对象的访问时间超过 5 分钟后会被删除。
3. 使用缓存
在 Java 代码中使用缓存,需要使用 SpringBoot 提供的 @Cacheable、@CachePut、@CacheEvict 注解。
例如,以下代码演示了如何使用 @Cacheable 注解:
```java
@Service
public class UserService {
@Cacheable(value = "userCache", key = "#id")
public User getUserById(Long id) {
// 从数据库中获取用户信息
User user = userDao.getUserById(id);
return user;
}
}
```
这里定义了一个缓存名为 userCache,并且根据 id 作为缓存的 key 值。
4. 测试缓存
在测试类中进行测试:
```java
@SpringBootTest
class UserServiceTest {
@Autowired
private UserService userService;
@Test
void testCache() {
// 第一次查询,从数据库中获取用户信息
User user1 = userService.getUserById(1L);
System.out.println(user1);
// 第二次查询,从缓存中获取用户信息
User user2 = userService.getUserById(1L);
System.out.println(user2);
}
}
```
这里定义了一个测试用例,第一次查询会从数据库中获取用户信息,第二次查询会从缓存中获取用户信息。
以上就是 SpringBoot 使用 Caffeine 缓存的教程,希望能对你有所帮助。
阅读全文
相关推荐
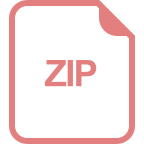
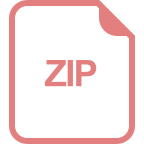












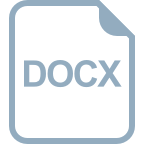
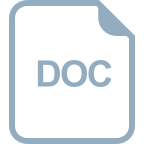


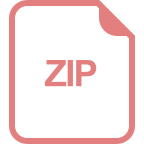