基于stm32的智能鱼缸自动温控代码
时间: 2023-08-31 07:13:01 浏览: 164
以下是一个基于STM32的智能鱼缸自动温控代码的示例:
```c
#include "stm32f10x.h"
#include "stdio.h"
#include "math.h"
//定义DS18B20引脚
#define DS18B20_PIN GPIO_Pin_0
#define DS18B20_PORT GPIOA
//定义继电器引脚
#define RELAY_PIN GPIO_Pin_1
#define RELAY_PORT GPIOA
//定义温度阈值
#define TEMP_THRESHOLD 25.0
//延时函数
void Delay_us(uint32_t nus)
{
uint32_t i;
for(i = 0; i < nus; i++)
{
__NOP();
}
}
//DS18B20复位函数
uint8_t DS18B20_Reset(void)
{
uint8_t i;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = DS18B20_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
GPIO_ResetBits(DS18B20_PORT, DS18B20_PIN);
Delay_us(480);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
Delay_us(80);
if(GPIO_ReadInputDataBit(DS18B20_PORT, DS18B20_PIN))
{
return 0;
}
Delay_us(400);
if(!GPIO_ReadInputDataBit(DS18B20_PORT, DS18B20_PIN))
{
return 0;
}
while(GPIO_ReadInputDataBit(DS18B20_PORT, DS18B20_PIN));
return 1;
}
//DS18B20写位函数
void DS18B20_WriteBit(uint8_t bit)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = DS18B20_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
if(bit)
{
GPIO_ResetBits(DS18B20_PORT, DS18B20_PIN);
Delay_us(5);
GPIO_SetBits(DS18B20_PORT, DS18B20_PIN);
Delay_us(90);
}
else
{
GPIO_ResetBits(DS18B20_PORT, DS18B20_PIN);
Delay_us(90);
GPIO_SetBits(DS18B20_PORT, DS18B20_PIN);
Delay_us(5);
}
}
//DS18B20写字节函数
void DS18B20_WriteByte(uint8_t byte)
{
uint8_t i;
for(i = 0; i < 8; i++)
{
DS18B20_WriteBit(byte & 0x01);
byte >>= 1;
}
}
//DS18B20读位函数
uint8_t DS18B20_ReadBit(void)
{
uint8_t bit;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = DS18B20_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
GPIO_ResetBits(DS18B20_PORT, DS18B20_PIN);
Delay_us(2);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
Delay_us(10);
bit = GPIO_ReadInputDataBit(DS18B20_PORT, DS18B20_PIN);
Delay_us(50);
return bit;
}
//DS18B20读字节函数
uint8_t DS18B20_ReadByte(void)
{
uint8_t i;
uint8_t byte = 0;
for(i = 0; i < 8; i++)
{
byte >>= 1;
if(DS18B20_ReadBit())
{
byte |= 0x80;
}
}
return byte;
}
//DS18B20读温度函数
float DS18B20_ReadTemp(void)
{
uint8_t temp[2];
float val;
DS18B20_Reset();
DS18B20_WriteByte(0xCC);
DS18B20_WriteByte(0x44);
Delay_us(750000);
DS18B20_Reset();
DS18B20_WriteByte(0xCC);
DS18B20_WriteByte(0xBE);
temp[0] = DS18B20_ReadByte();
temp[1] = DS18B20_ReadByte();
val = (float)(temp[1] << 8 | temp[0]) * 0.0625;
return val;
}
//继电器控制函数
void Relay_Control(uint8_t state)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = RELAY_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(RELAY_PORT, &GPIO_InitStructure);
if(state)
{
GPIO_SetBits(RELAY_PORT, RELAY_PIN);
}
else
{
GPIO_ResetBits(RELAY_PORT, RELAY_PIN);
}
}
int main(void)
{
float temp;
while(1)
{
temp = DS18B20_ReadTemp();
if(temp > TEMP_THRESHOLD)
{
Relay_Control(1);
}
else
{
Relay_Control(0);
}
}
}
```
此代码使用DS18B20传感器读取温度,如果温度高于设定的阈值,就会控制继电器开启风扇或者制冷器等来降低温度。请注意,在使用此代码之前,需要对STM32的GPIO进行初始化和配置。
阅读全文
相关推荐
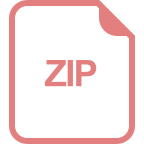


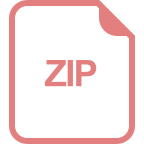



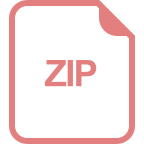
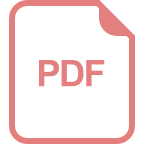
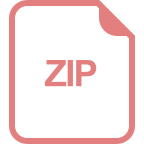
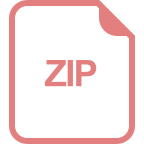
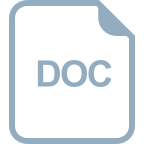



