java把数据拆分成多个消息通过消息中间件发送,完整接收同一批消息,代码如何编写
时间: 2024-09-26 21:14:44 浏览: 34
在Java中,通常会使用像Apache ActiveMQ、RabbitMQ或Kafka这样的消息中间件来实现异步通信和消息的可靠传输。这里以RabbitMQ为例说明:
首先,你需要设置好RabbitMQ服务器,并创建一个生产者(Producer),它负责将数据拆分为消息并发送到队列:
```java
import com.rabbitmq.client.ConnectionFactory;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.Channel;
public class Producer {
private static final String QUEUE_NAME = "your_queue_name";
public void sendMessage(String data) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost"); // 修改为你实际的RabbitMQ服务器地址
Connection connection = factory.newConnection();
Channel channel = connection.createChannel();
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
String message = "[" + data + "]";
channel.basicPublish("", QUEUE_NAME, null, message.getBytes("UTF-8"));
System.out.println(" [x] Sent '" + message + "'");
channel.close();
connection.close();
}
}
```
然后,在消费者(Consumer)端,你需要订阅该队列并处理接收到的消息,确保能接收到同一批消息并进行完整的处理:
```java
import com.rabbitmq.client.ConnectionFactory;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.DeliverCallback;
public class Consumer {
private static final String QUEUE_NAME = "your_queue_name";
public void consumeMessage(DeliverCallback deliverCallback) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost"); // 修改为你实际的RabbitMQ服务器地址
Connection connection = factory.newConnection();
Channel channel = connection.createChannel();
channel.basicConsume(QUEUE_NAME, true, deliverCallback, consumerTag -> {/* do nothing */}, consumerProperties -> {/* do nothing */});
channel.basicAck(deliverCallback.getEnvelope().getDeliveryTag(), false); // 消费确认,防止消息丢失
connection.addShutdownListener(channel::close); // 关闭连接时,自动关闭channel
connection.waitForShutdownOrClose();
}
// 定义回调函数来处理接收到的消息
private void handleDelivery(String routingKey, byte[] body, Channel ch) {
String message = new String(body, "UTF-8");
System.out.println(" [.] Received '" + message + "'");
// 这里处理接收到的数据,例如存储到数据库或进一步操作
}
}
```
最后,你可以创建`DeliverCallback`实例并将`handleDelivery`作为回调函数传递给消费者:
```java
DeliverCallback callback = (consumerTag, delivery) -> {
try {
handleDelivery(delivery.getEnvelope().getRoutingKey(), delivery.getBody(), delivery.getChannel());
} catch (Exception e) {
// 错误处理
e.printStackTrace();
}
};
new Consumer().consumeMessage(callback);
```
阅读全文
相关推荐
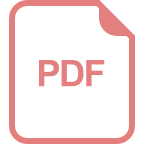
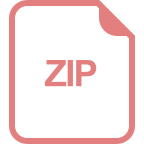
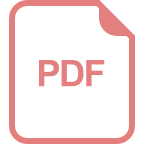

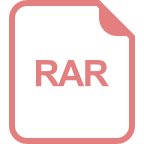
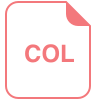
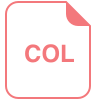
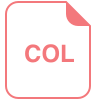
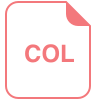
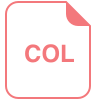
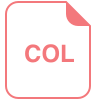
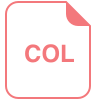
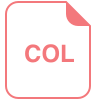
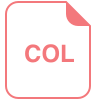
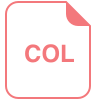
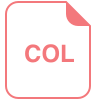
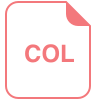
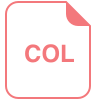
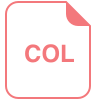