如何在C#服务中持久保存登录凭据?
时间: 2024-10-09 07:09:58 浏览: 46
在C# Windows服务中持久保存登录凭据,通常不建议直接将明文密码存储在代码中,因为这会增加安全性风险。一种常见的做法是在本地存储如Windows用户账户凭据或者是应用程序数据文件中使用.NET Framework的安全措施来管理。
**使用`System.Security.Cryptography.ProtectedData`**:
1. 将密码转换为`byte[]`数组。
2. 使用`Protect`方法将其加密,并指定保护算法(如`DataProtectionMethod.LocalMachine`)。
3. 存储加密后的数据,比如在XML文件或专用的数据存储库(如`.config`文件)中。
```csharp
using System.Security.Cryptography;
using System.Configuration;
string password = "your_password";
byte[] encryptedPassword = ProtectedData.Protect(Encoding.UTF8.GetBytes(password), null, DataProtectionScope.LocalMachine);
// 存储加密密码
string configKey = "CredentialKey";
ConfigurationManager.AppSettings[configKey] = Convert.ToBase64String(encryptedPassword);
```
当需要使用凭据时,执行相反的操作:
```csharp
byte[] decryptedPassword = Convert.FromBase64String(ConfigurationManager.AppSettings[configKey]);
string password = Encoding.UTF8.GetString(ProtectedData.Unprotect(decryptedPassword, null, DataProtectionScope.LocalMachine));
```
**使用WindowsCredential类**:
Windows系统提供了一种更底层的方式来保存和获取凭据,`System.Security.Authentication\CredentialCache`。这个方法更适合跨域认证,例如NTLM。
```csharp
var store = new CredentialCache();
store.Add(new Uri(serverUri), "Negotiate", new NetworkCredential("username", password));
CredentialManager.CredentialVault.Add(store);
```
记得在每次需要访问时从`CredentialManager`中检索凭据。
阅读全文
相关推荐
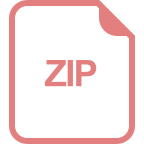
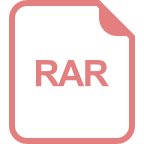
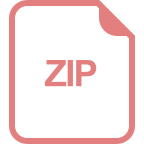
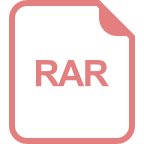
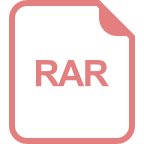
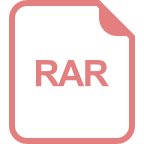
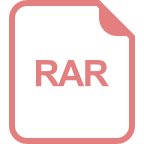
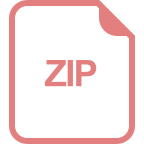
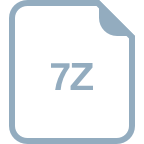
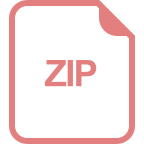
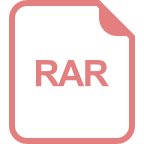
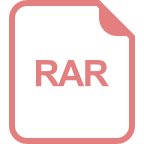
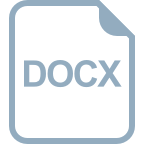
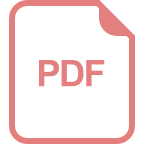
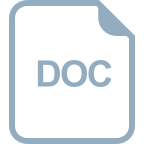
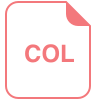
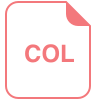
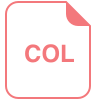
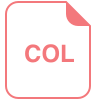