用 c++写一个单链表冒泡排序
时间: 2024-05-08 12:18:01 浏览: 7
Sure, here's an example code for bubble sort on a singly linked list using C:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void swap(struct Node* a, struct Node* b) {
int temp = a->data;
a->data = b->data;
b->data = temp;
}
void sortList(struct Node* head) {
int swapped, i;
struct Node* ptr1;
struct Node* lptr = NULL;
if (head == NULL)
return;
do {
swapped = 0;
ptr1 = head;
while (ptr1->next != lptr) {
if (ptr1->data > ptr1->next->data) {
swap(ptr1, ptr1->next);
swapped = 1;
}
ptr1 = ptr1->next;
}
lptr = ptr1;
} while (swapped);
}
void printList(struct Node* head) {
struct Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
void push(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
int main() {
struct Node* head = NULL;
push(&head, 1);
push(&head, 4);
push(&head, 3);
push(&head, 2);
printf("Unsorted list: ");
printList(head);
sortList(head);
printf("Sorted list: ");
printList(head);
return 0;
}
```
Note: This implementation uses the basic bubble sort algorithm with a runtime complexity of O(n^2). There are faster sorting algorithms available for linked lists, but this should suffice for small lists.
相关推荐
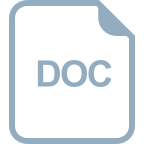









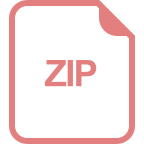
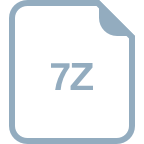
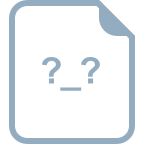
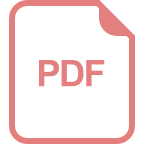