Esp8266和ht66f2390代码
时间: 2024-04-14 15:19:12 浏览: 246
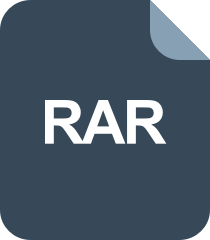
代码esp8266
对于ESP8266,这里提供一个简单的示例代码,可以通过Wi-Fi连接到Internet,并向指定的URL发送HTTP请求:
```c
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
void setup() {
Serial.begin(9600);
delay(10);
// Connect to WiFi network
Serial.println();
Serial.println();
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
void loop() {
WiFiClient client;
const int httpPort = 80;
if (!client.connect("www.example.com", httpPort)) {
Serial.println("connection failed");
return;
}
// We now create a URI for the request
String url = "/api/v1/data";
Serial.print("Requesting URL: ");
Serial.println(url);
// This will send the request to the server
client.print(String("GET ") + url + " HTTP/1.1\r\n" +
"Host: www.example.com\r\n" +
"Connection: close\r\n\r\n");
delay(500);
// Read all the lines of the response from server and print them to Serial
while(client.available()){
String line = client.readStringUntil('\r');
Serial.print(line);
}
Serial.println();
Serial.println("closing connection");
}
```
对于HT66F2390,这里提供一个简单的示例代码,可以使用定时器中断来控制LED灯的闪烁:
```c
#include "ht66f2390.h"
// Define LED pin
sbit LED = P3^5;
void Timer0_ISR() interrupt 1
{
static unsigned char led_state = 0;
// Toggle LED state
led_state = !led_state;
LED = led_state;
}
void main()
{
// Initialize timer0
TMOD = 0x01; // Set timer0 as 16-bit timer
TH0 = 0xFC; // Set timer0 initial value
TL0 = 0x67;
TR0 = 1; // Enable timer0
ET0 = 1; // Enable timer0 interrupt
// Enable interrupts
EA = 1;
while(1)
{
// Main loop
}
}
```
这是一个简单的例子,使用定时器中断控制LED灯的闪烁。在Timer0_ISR()函数中,每次中断时,改变LED灯的状态,并在主循环中等待。请注意,这只是一个简单的示例代码,可能需要根据您的具体应用进行修改。
阅读全文
相关推荐
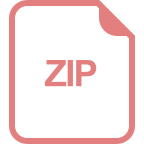
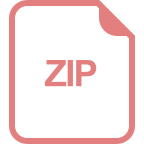
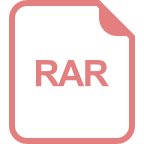
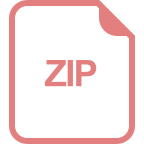
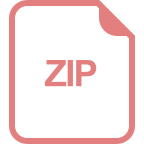
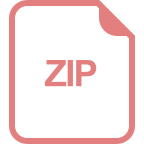
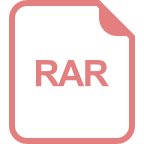
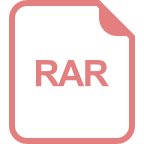
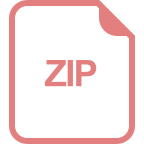
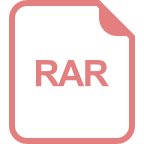
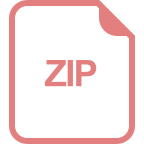
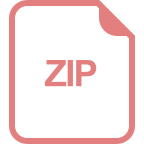
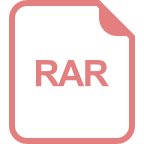
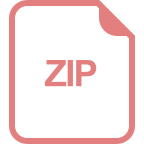