使用js制作一个在线考试系统:
时间: 2024-03-15 17:45:06 浏览: 83
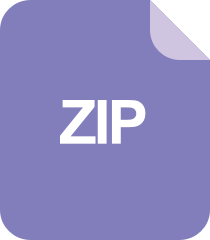
OnlineExamination.zip_BF-522在线_django在线考试_fffb&info_onlineexamin
好的,那我来给您介绍一下如何使用 JavaScript 制作一个在线考试系统。
首先,我们需要确定考试的题目类型和数量,然后将这些题目存储在一个数组或对象中。比如,我们可以定义一个存储选择题的数组:
```javascript
var questions = [
{
question: "What is the capital of France?",
choices: ["London", "Paris", "New York", "Tokyo"],
correctAnswer: 1
},
{
question: "What is the largest planet in our solar system?",
choices: ["Saturn", "Jupiter", "Mercury", "Neptune"],
correctAnswer: 1
},
{
question: "What is the tallest mountain in the world?",
choices: ["Mount Kilimanjaro", "Mount Everest", "Mount Fuji", "Mount Whitney"],
correctAnswer: 1
}
];
```
然后,我们需要编写一个函数来显示每个问题和它们的选项。这个函数可以在 HTML 页面中创建一些元素(例如,`<div>`、`<p>`、`<ul>` 和 `<li>`),并将问题和选项插入到这些元素中。然后,当用户选择一个答案时,我们可以检查它是否正确,并将用户的得分存储在一个变量中。
```javascript
var score = 0;
function displayQuestion(question) {
var questionContainer = document.createElement("div");
questionContainer.innerHTML = "<p>" + question.question + "</p>";
var choicesList = document.createElement("ul");
for (var i = 0; i < question.choices.length; i++) {
var choiceItem = document.createElement("li");
var choiceText = document.createTextNode(question.choices[i]);
choiceItem.appendChild(choiceText);
choiceItem.onclick = function() {
if (i === question.correctAnswer) {
score++;
}
nextQuestion();
};
choicesList.appendChild(choiceItem);
}
questionContainer.appendChild(choicesList);
document.body.appendChild(questionContainer);
}
```
最后,我们需要编写一个函数来遍历问题数组,并显示每个问题。这个函数也可以在 HTML 页面中创建一个“下一题”按钮,当用户点击该按钮时,它会显示下一个问题。
```javascript
var currentQuestion = 0;
function nextQuestion() {
currentQuestion++;
if (currentQuestion < questions.length) {
document.body.innerHTML = "";
displayQuestion(questions[currentQuestion]);
} else {
document.body.innerHTML = "<p>Your score is " + score + " out of " + questions.length + ".</p>";
}
}
displayQuestion(questions[currentQuestion]);
```
这样,我们就完成了一个简单的 JavaScript 在线考试系统。当用户完成所有问题后,它会显示用户的得分。当然,这只是一个简单的示例,您可以根据需要对它进行修改和扩展。
阅读全文
相关推荐
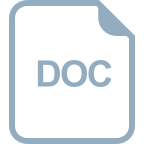
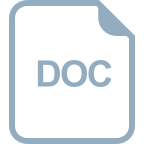
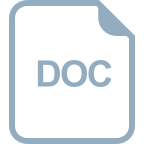
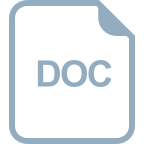
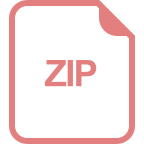
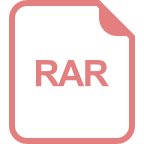
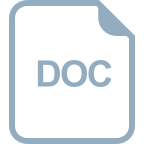
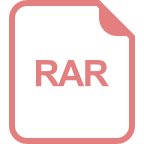
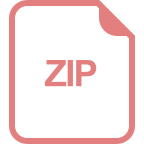
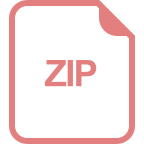
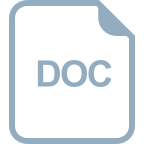
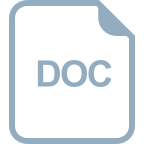
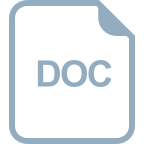
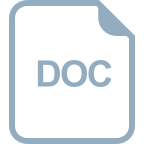
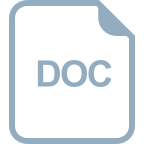
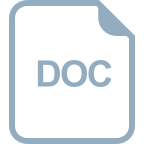

