java zip中嵌套zip解压
时间: 2023-07-01 09:25:02 浏览: 223
Java自带的ZipInputStream类可以用于解压缩zip文件,而对于嵌套的zip文件,我们可以通过递归调用ZipInputStream来实现解压缩。
以下是一个示例代码,用于解压缩一个包含嵌套zip文件的zip文件:
```java
import java.io.*;
import java.util.zip.*;
public class NestedZipExtractor {
public static void main(String[] args) throws IOException {
String zipFilePath = "path/to/nested/zip/file.zip";
String outputFolder = "path/to/output/folder";
unzipNestedZipFiles(zipFilePath, outputFolder);
}
public static void unzipNestedZipFiles(String zipFilePath, String outputFolder) throws IOException {
File outputDir = new File(outputFolder);
if (!outputDir.exists()) {
outputDir.mkdir();
}
FileInputStream fis = new FileInputStream(zipFilePath);
ZipInputStream zis = new ZipInputStream(fis);
ZipEntry zipEntry = zis.getNextEntry();
byte[] buffer = new byte[1024];
while (zipEntry != null) {
String fileName = zipEntry.getName();
File newFile = new File(outputFolder + File.separator + fileName);
if (zipEntry.isDirectory()) {
newFile.mkdirs();
} else {
FileOutputStream fos = new FileOutputStream(newFile);
int len;
while ((len = zis.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
fos.close();
// 如果解压出来的是一个zip文件,则递归调用解压函数
if (fileName.endsWith(".zip")) {
unzipNestedZipFiles(newFile.getAbsolutePath(), outputFolder + File.separator + fileName.replace(".zip", ""));
}
}
zis.closeEntry();
zipEntry = zis.getNextEntry();
}
zis.close();
}
}
```
这段代码会递归地解压缩所有嵌套的zip文件,并将解压后的文件保存到指定的输出文件夹中。注意,虽然这段代码处理了嵌套的zip文件,但是它并没有处理嵌套的压缩文件格式,如tar.gz等。如果需要处理这些格式的文件,可以根据需要进行扩展。
阅读全文
相关推荐

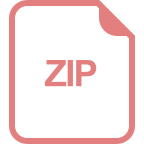
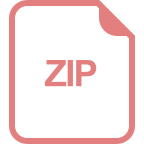
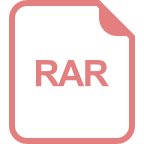
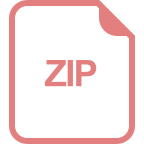
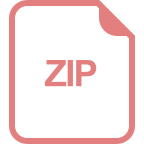
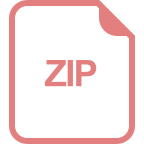
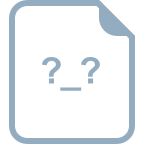
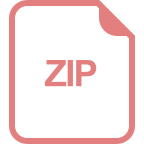
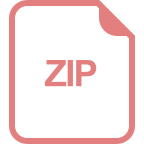
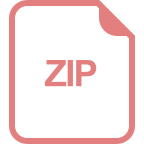
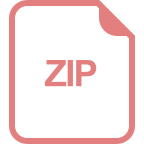
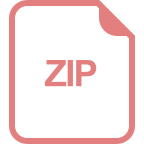
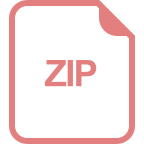
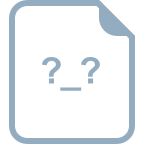
