用Julia语言编写完整程序,计算炮弹飞行轨迹及瞬时速度,炮弹的初速度和发射角度为输入参数,考虑有、无空气阻力两种情况,并将结果画图。
时间: 2024-11-11 13:36:47 浏览: 18
当然,下面是使用Julia编写的一个完整的程序,它包含了计算炮弹飞行轨迹和瞬时速度的函数,并在无空气阻力和有空气阻力的情况下进行了对比。这个例子假设炮弹的质量不变,只考虑了水平方向的运动。
```julia
# 引入需要的库
using Plots
using DifferentialEquations
# 定义炮弹运动的函数,空气阻力系数为可选参数
function ballistic_motion(speed, angle, gravity, drag_coefficient = 0.0)
@unpack vx0, vy0, m = decompose_speed(speed, angle)
# 没有空气阻力的情况
function no_drag(t, state)
[vx0 + gravity*t*sin(angle)/m, vy0 - gravity*t*cos(angle)]
end
# 考虑空气阻力的情况
function with_drag(t, state)
[vx0 + gravity*t*sin(angle)/m - drag_coefficient*state[1]*sin(angle), vy0 - gravity*t*cos(angle)]
end
# 分别计算两种情况下的轨迹
no_drag_solution = solve(ODEProblem(no_drag, [vx0, vy0], (0., 10.), (speed, speed, m)), Tsit5())
with_drag_solution = solve(ODEProblem(with_drag, [vx0, vy0], (0., 10.), (speed, speed, m)), Tsit5())
# 提取位置和速度数据
no_drag_positions = no_drag_solution.states[:, 1]
no_drag_velocities = no_drag_solution.states[:, 2]
with_drag_positions = with_drag_solution.states[:, 1]
with_drag_velocities = with_drag_solution.states[:, 2]
return no_drag_positions, no_drag_velocities, with_drag_positions, with_drag_velocities
end
# 函数分解速度到水平和垂直方向的速度
function decompose_speed(speed, angle)
vx0 = speed * cos(angle)
vy0 = speed * sin(angle)
m = speed # 这里我们简单假设炮弹质量与速度成正比,实际应用中应考虑真实质量
return vx0, vy0, m
end
# 主函数绘制图形
function main(speed, angle, drag_coefficient = 0.0)
positions_no_drag, velocities_no_drag, positions_with_drag, velocities_with_drag = ballistic_motion(speed, angle, 9.81, drag_coefficient)
# 绘制飞行轨迹
p1 = plot(positions_no_drag, label="无空气阻力轨迹", lw=2)
plot!(positions_with_drag, label="有空气阻力轨迹", lw=2, ls=:dash)
# 绘制瞬时速度
p2 = plot(velocities_no_drag, label="无空气阻力速度", lw=2)
plot!(velocities_with_drag, label="有空气阻力速度", lw=2, ls=:dash)
layout([p1, p2])
display(plot(p1, p2, size = (800, 600)))
end
# 示例调用
main(1000, π/4)
```
在这个程序中,用户可以通过调用`main`函数并传入初速度和发射角来查看两种情况下炮弹的轨迹和瞬时速度。注意,这里的空气阻力简化处理了,实际应用中可能需要考虑更为复杂的阻力模型。
阅读全文
相关推荐
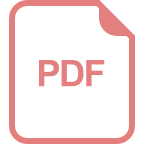
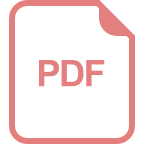
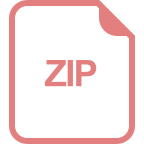

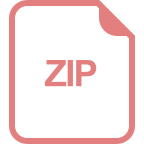
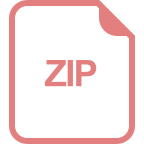
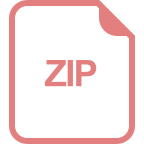
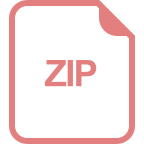
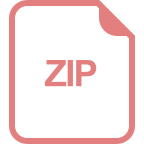
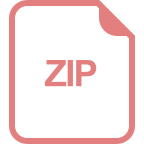
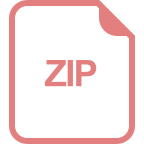
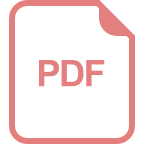
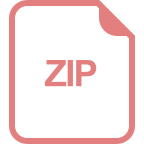
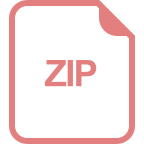
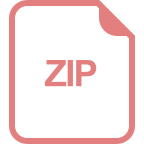
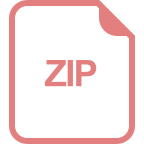
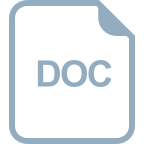
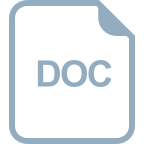
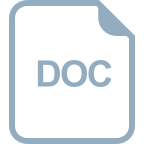
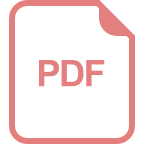