C#检索文件夹中所有的“.jpg”文件并按生成时间排序生成List<string>变量
时间: 2024-09-14 15:16:15 浏览: 46
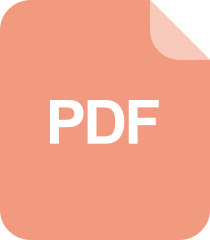
C# List介绍及具体用法

在C#中,您可以使用`System.IO`命名空间下的`Directory`和`FileInfo`类来检索指定文件夹中所有的“.jpg”文件并按生成时间排序。以下是一个示例代码:
```csharp
using System;
using System.IO;
using System.Collections.Generic;
class Program
{
static void Main()
{
string folderPath = @"C:\path\to\your\folder"; // 指定文件夹路径
string searchPattern = "*.jpg"; // 指定搜索模式为所有的.jpg文件
// 获取所有.jpg文件的文件信息
FileInfo[] files = Directory.GetFiles(folderPath, searchPattern, SearchOption.TopDirectoryOnly)
.Select(path => new FileInfo(path))
.ToArray();
// 按生成时间排序
Array.Sort(files, (x, y) => x.LastWriteTime.CompareTo(y.LastWriteTime));
// 将排序后的文件路径添加到List中
List<string> sortedJpgFiles = new List<string>();
foreach (FileInfo file in files)
{
sortedJpgFiles.Add(file.FullName);
}
// 输出结果
foreach (string filePath in sortedJpgFiles)
{
Console.WriteLine(filePath);
}
}
}
```
在这段代码中,首先通过`Directory.GetFiles`方法获取指定路径下所有匹配模式的文件路径数组。然后,使用`Select`方法将这些路径转换为`FileInfo`对象数组,因为`FileInfo`类提供了`LastWriteTime`属性,可以用来获取文件的最后修改时间。接着,使用`Array.Sort`方法并传入一个比较器来根据最后写入时间对文件进行排序。最后,通过遍历排序后的`FileInfo`数组,将每个文件的完整路径添加到`List<string>`中。
阅读全文
相关推荐
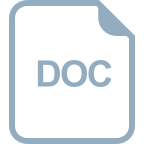
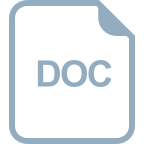
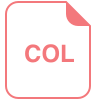
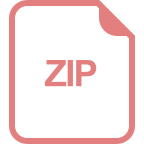
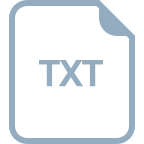
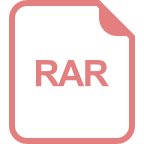
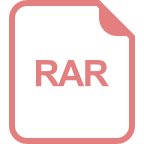
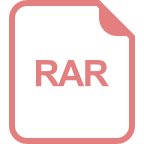
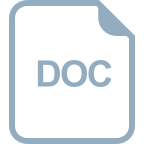
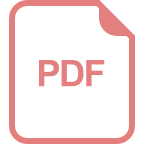
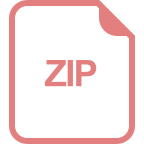
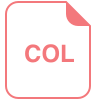
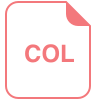
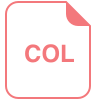
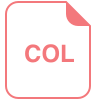
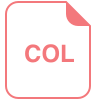
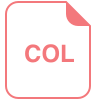
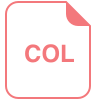