用Python或者c++实现A*
时间: 2024-11-10 16:27:15 浏览: 8
在Python中实现A*算法,我们可以使用内置的数据结构,如列表和字典,配合优先级队列(例如heapq模块)。以下是基本的Python代码实现:
```python
import heapq
# 定义一个表示节点的数据结构
class AStarNode:
def __init__(self, pos, cost=0, parent=None):
self.pos = pos
self.cost = cost
self.parent = parent
self.g = cost
self.h = heuristic_function(self.pos, target_pos) # 假设heuristic_function是你自定义的估价函数
self.f = self.g + self.h
def a_star_search(graph, start, target):
open_list = [(start.f, start)]
closed_list = set()
while open_list:
_, current = heapq.heappop(open_list)
if current.pos == target:
return reconstruct_path(current)
if current in closed_list:
continue
closed_list.add(current)
for neighbor in graph.neighbors(current.pos): # 假设graph.neighbors获取邻接节点
tentative_g = current.cost + graph.cost(current.pos, neighbor.pos)
if neighbor not in open_list or tentative_g < neighbor.g:
neighbor.g = tentative_g
neighbor.parent = current
neighbor.h = heuristic_function(neighbor.pos, target_pos)
neighbor.f = neighbor.g + neighbor.h
heapq.heappush(open_list, (neighbor.f, neighbor))
return None # 如果找不到路径,则返回None
# 辅助函数,重建路径
def reconstruct_path(end_node):
path = [end_node]
while end_node.parent:
end_node = end_node.parent
path.append(end_node)
return list(reversed(path))
```
对于C++,你可以使用STL库来实现类似的功能:
```cpp
#include <queue>
#include <unordered_set>
struct AStarNode {
std::pair<int, int> position;
int cost;
AStarNode *parent;
int g, h, f;
AStarNode(const std::pair<int, int>& pos, int initial_cost = 0, AStarNode *parent_ = nullptr)
: position(pos), cost(initial_cost), parent(parent_), g(initial_cost), h(h_value(position)), f(g+h)
{}
bool operator<(const AStarNode& other) const {
return f > other.f;
}
};
std::vector<std::pair<int, int>> a_star_search(std::function<std::vector<AStarNode>(const std::pair<int, int>)>& neighbors, const AStarNode& start, const AStarNode& target) {
std::priority_queue<AStarNode, std::vector<AStarNode>, std::greater<AStarNode>> open_list;
std::unordered_set<AStarNode*> closed_list;
open_list.push(start);
while (!open_list.empty()) {
auto current = open_list.top(); open_list.pop();
if (current.position == target.position) {
return reconstruct_path(current);
}
if (closed_list.count(¤t)) continue;
closed_list.insert(¤t);
for (const auto& neighbor : neighbors(current.position)) {
int tentative_g = current.cost + neighbor.cost; // 假设cost函数返回两个节点之间的代价
if (!closed_list.count(&neighbor) || tentative_g < neighbor.g) {
neighbor.g = tentative_g;
neighbor.parent = ¤t;
neighbor.h = h_value(neighbor.position); // 假设h_value是估价函数
neighbor.f = neighbor.g + neighbor.h;
open_list.push(neighbor);
}
}
}
return {}; // 如果找不到路径,返回空向量
}
// 辅助函数,重构路径
std::vector<std::pair<int, int>> reconstruct_path(const AStarNode& end_node) {
std::vector<std::pair<int, int>> path;
while (end_node.parent) {
path.push_back(end_node.position);
end_node = *end_node.parent;
}
return path;
}
```
别忘了根据你的需求调整`heuristic_function`、`neighbors`、`h_value`等部分。
阅读全文
相关推荐
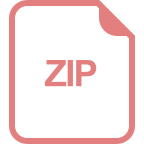
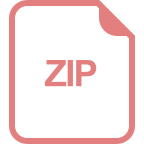
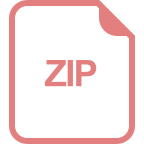
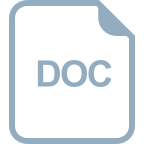


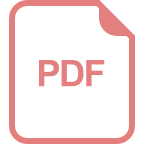
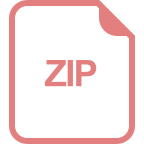
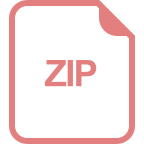
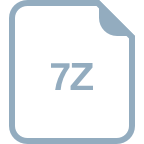
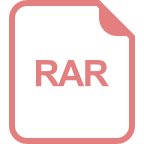
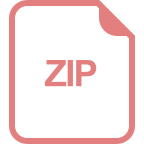
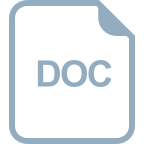
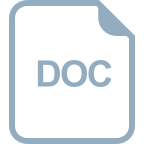
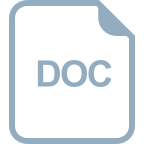
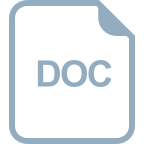



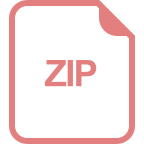