python将图片批量判断单元格和图片名字是否一致,一致则写入excel表格
时间: 2024-11-12 21:39:33 浏览: 16
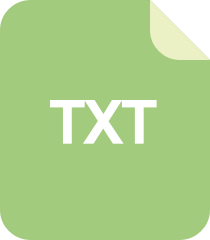
python-批量处理指定文件夹下的excel文档指定单元格内容
在Python中,你可以使用Pandas库处理Excel数据,OpenCV或PIL库读取和操作图片,以及os模块来遍历文件夹。下面是一个简单的流程:
1. 首先,安装必要的库,如`pandas`, `numpy`, `opencv-python-headless` 或 `Pillow`(如果你还没安装的话):
```bash
pip install pandas numpy opencv-python-headless pillow
```
2. 使用os模块获取图片目录及其名称,然后读取每个图片到图像数组中:
```python
import os
import cv2
from PIL import Image
image_dir = 'path/to/image/directory'
images = [cv2.imread(os.path.join(image_dir, filename)) for filename in os.listdir(image_dir)]
```
3. 对于每个图片,你需要将其转换成可以比较的文字描述,例如使用OCR(光学字符识别)技术,这里以Tesseract为例:
```python
import pytesseract
pytesseract.pytesseract.tesseract_cmd = r'C:\Program Files\Tesseract-OCR\tesseract.exe' # 设置Tesseract路径
text_descriptions = [pytesseract.image_to_string(Image.fromarray(img)) for img in images]
```
4. 然后打开Excel并创建一个DataFrame,其中一列对应图片名,另一列对应OCR提取的文字:
```python
import pandas as pd
df = pd.DataFrame({'Image Name': os.listdir(image_dir), ' OCR Text': text_descriptions})
```
5. 比较两个列,如果图片名和OCR文字一致,就将它们写入新的Excel文件:
```python
def check_and_write(row):
if row['Image Name'] == row[' OCR Text']:
return True
else:
return False
df['Match'] = df.apply(check_and_write, axis=1)
matched_df = df[df['Match']]
matched_df.to_excel('matched_data.xlsx', index=False)
```
阅读全文
相关推荐
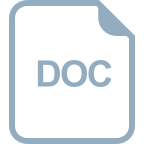
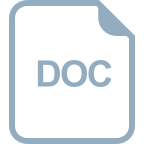
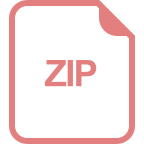
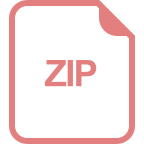
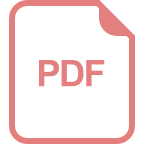
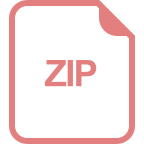
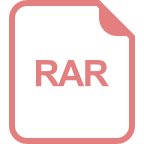
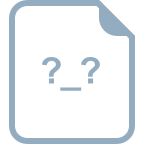
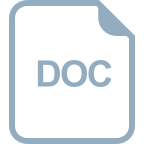
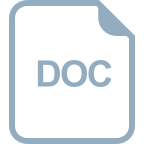
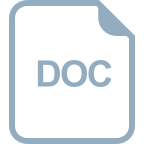
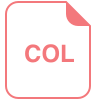





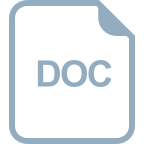