使用hashmap实现一个简单的图书管理系统,包含添加,删除,查询书籍的功能(书名与作者)的Java代码
时间: 2024-09-27 13:05:10 浏览: 21
在Java中,我们可以使用HashMap来实现一个简单的图书管理系统,因为HashMap提供了快速查找、插入和删除元素的能力,非常适合用于这种键值对的操作。以下是一个基本的实现:
```java
import java.util.HashMap;
class Book {
String title;
String author;
public Book(String title, String author) {
this.title = title;
this.author = author;
}
@Override
public String toString() {
return "Book{" +
"title='" + title + '\'' +
", author='" + author + '\'' +
'}';
}
}
public class LibrarySystem {
private HashMap<String, Book> library;
public LibrarySystem() {
library = new HashMap<>();
}
// 添加书籍
public void addBook(String title, String author) {
Book book = new Book(title, author);
library.put(title, book);
System.out.println("Book added: " + book);
}
// 删除书籍(如果存在)
public boolean removeBook(String title) {
if (library.containsKey(title)) {
library.remove(title);
System.out.println("Book removed: " + title);
return true;
} else {
System.out.println("Book not found.");
return false;
}
}
// 查询书籍
public Book searchBook(String title) {
return library.get(title);
}
public static void main(String[] args) {
LibrarySystem system = new LibrarySystem();
system.addBook("The Great Gatsby", "F. Scott Fitzgerald");
system.addBook("To Kill a Mockingbird", "Harper Lee");
Book bookFound = system.searchBook("To Kill a Mockingbird");
if (bookFound != null) {
System.out.println("Found book: " + bookFound);
} else {
System.out.println("Book not found.");
}
system.removeBook("The Great Gatsby");
}
}
```
在这个例子中,`LibrarySystem`类有一个HashMap,存储了书名到Book对象的映射。添加书籍通过标题插入,删除书籍根据标题查找并移除,查询书籍则直接返回对应标题的Book对象。在main函数中演示了如何操作这个系统。
相关推荐
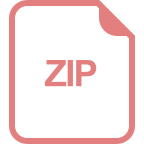
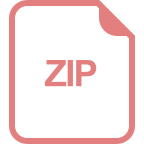
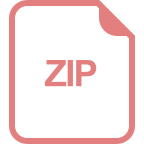














