SpringBoot集成ffmpeg
时间: 2023-08-18 17:08:37 浏览: 106
在Spring Boot中集成FFmpeg可以实现视频处理、转码、剪辑等功能。下面是一个简单的步骤:
1. 首先,你需要将FFmpeg库添加到你的项目中。你可以通过以下几种方式之一来完成:
- 将FFmpeg可执行文件(例如ffmpeg.exe)添加到你的项目的资源文件夹中。
- 使用Maven或Gradle等构建工具,在你的项目的依赖管理文件中添加FFmpeg的依赖。
2. 创建一个Java类,用于调用FFmpeg命令行工具。你可以使用Java的ProcessBuilder类来执行命令行操作。下面是一个简单的示例:
```java
import java.io.IOException;
public class FFmpegUtils {
public static void convertVideo(String inputPath, String outputPath) throws IOException {
// 构建FFmpeg命令行
String[] command = {"ffmpeg", "-i", inputPath, outputPath};
// 执行命令行
ProcessBuilder processBuilder = new ProcessBuilder(command);
Process process = processBuilder.start();
// 等待命令执行完成
try {
process.waitFor();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
3. 在你的Spring Boot应用程序中,调用上述方法来执行视频转码操作。你可以在Controller层或Service层中调用该方法。
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
@RestController
public class VideoController {
@PostMapping("/convert")
public String convertVideo(@RequestParam("input") String inputPath, @RequestParam("output") String outputPath) {
try {
FFmpegUtils.convertVideo(inputPath, outputPath);
return "转码成功!";
} catch (IOException e) {
e.printStackTrace();
return "转码失败!";
}
}
}
```
以上示例中的`convertVideo`方法可以将输入视频文件转码为输出视频文件。你可以根据需要添加其他FFmpeg命令行参数来实现不同的视频处理操作。
请注意,集成FFmpeg需要你的操作系统中已经安装了FFmpeg,并且可执行文件在系统的环境变量中可用。另外,FFmpeg是一个功能强大而复杂的工具,你可能需要根据具体需求进行更多的配置和调整。
相关推荐
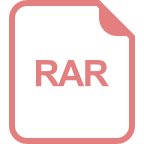
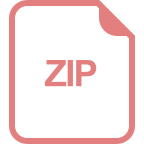
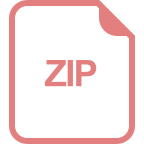














