用java实现多线程消费者和生产者模型
时间: 2023-03-19 22:22:22 浏览: 175
好的,以下是一个使用Java语言实现的生产者和消费者模型的示例:
```
import java.util.LinkedList;
public class ProducerConsumerExample {
public static void main(String[] args) {
LinkedList<Integer> queue = new LinkedList<>();
int maxSize = 5;
Producer producer = new Producer(queue, maxSize);
Consumer consumer = new Consumer(queue);
Thread producerThread = new Thread(producer, "Producer");
Thread consumerThread = new Thread(consumer, "Consumer");
producerThread.start();
consumerThread.start();
}
}
class Producer implements Runnable {
private LinkedList<Integer> queue;
private int maxSize;
public Producer(LinkedList<Integer> queue, int maxSize) {
this.queue = queue;
this.maxSize = maxSize;
}
@Override
public void run() {
while (true) {
synchronized (queue) {
while (queue.size() == maxSize) {
try {
System.out.println("队列已满,生产者等待消费...");
queue.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
int number = (int) (Math.random() * 100);
queue.add(number);
System.out.println("生产者生产: " + number);
queue.notifyAll();
}
}
}
}
class Consumer implements Runnable {
private LinkedList<Integer> queue;
public Consumer(LinkedList<Integer> queue) {
this.queue = queue;
}
@Override
public void run() {
while (true) {
synchronized (queue) {
while (queue.isEmpty()) {
try {
System.out.println("队列为空,消费者等待生产...");
queue.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
int number = queue.removeFirst();
System.out.println("消费者消费: " + number);
queue.notifyAll();
}
}
}
}
```
上述代码实现了一个简单的生产者和消费者模型,其中使用了一个线程安全的`LinkedList`队列作为生产者和消费者之间的缓冲区。在`Producer`和`Consumer`类中,`run()`方法被覆盖并实现了生产和消费的逻辑。
在生产者线程中,如果队列已满,则生产者线程将进入等待状态。当队列不满时,生产者线程将生成随机数并将其添加到队列中,并通过调用`notifyAll()`方法通知消费者线程可以消费了。在消费者线程中,如果队列为空,则消费者线程将进入等待状态。当队列不为空时,消费者线程将从队列中删除第一个元素,并通过调用`notifyAll()`方法通知生产者线程可以继续生产。
这种实现方式使用`synchronized`关键字确保在对队列进行修改时线程安全。此外,生产者和消费者线程之间的通信使用了`wait()`和`notifyAll()`方法,以确保生产者和消费者之间的协调。
相关推荐
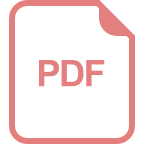
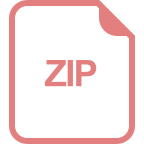














