springboot modelandview
时间: 2023-03-16 21:48:54 浏览: 167
Spring Boot中的ModelAndView是一个用于封装视图和模型数据的类。它可以将模型数据和视图名称一起传递给控制器方法,以便在视图中使用。在控制器方法中,可以使用ModelAndView的方法来设置模型数据和视图名称。最后,控制器方法将ModelAndView对象返回给Spring MVC框架,以便它可以将模型数据和视图渲染到客户端。
相关问题
freemarker springboot ModelAndView
Freemarker is a template engine that allows you to generate dynamic HTML pages. Spring Boot is a framework that simplifies the development of web applications by providing pre-configured settings and dependencies. ModelAndView is a class in Spring Boot that represents a model and view, used to pass data to a view and specify the view to be rendered.
To use Freemarker with Spring Boot, you need to add the following dependencies to your project:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
```
Next, you can create a controller class with a request mapping that returns a ModelAndView object:
```
@Controller
public class MyController {
@RequestMapping("/myPage")
public ModelAndView myPage() {
ModelAndView modelAndView = new ModelAndView("myPage");
modelAndView.addObject("message", "Hello World!");
return modelAndView;
}
}
```
In this example, the "myPage" template is used to render the view, and the "message" object is passed as a parameter to the template. The "message" object can be accessed in the template using the syntax ${message}.
Finally, you need to create the "myPage" template in the "templates" directory of your application. Here's an example of what the template might look like:
```
<!DOCTYPE html>
<html>
<head>
<title>My Page</title>
</head>
<body>
<h1>${message}</h1>
</body>
</html>
```
When the /myPage endpoint is accessed, Spring Boot will render the "myPage" template with the "Hello World!" message.
springboot使用modelandview全过程
Spring Boot使用ModelAndView来返回数据和视图,它是一个包含模型数据和视图名称的对象。ModelAndView将模型数据添加到模型中,并指定要返回的视图名称。
以下是使用ModelAndView的全过程:
1. 创建Controller类并添加@RequestMapping注解,该注解指定了请求的URL和HTTP方法。
2. 在Controller方法中创建ModelAndView对象。
3. 添加模型数据到ModelAndView对象中。
4. 设置视图名称,将其返回给DispatcherServlet。
5. DispatcherServlet将视图名称解析为视图对象,并使用模型数据渲染视图。
6. 渲染后的视图作为HTTP响应发送给客户端。
下面是一个示例Controller类,它返回一个包含“hello”消息的ModelAndView对象:
```
@Controller
public class ExampleController {
@RequestMapping(value = "/hello", method = RequestMethod.GET)
public ModelAndView hello() {
ModelAndView modelAndView = new ModelAndView();
modelAndView.addObject("message", "hello");
modelAndView.setViewName("hello");
return modelAndView;
}
}
```
在上面的示例中,我们创建了一个ModelAndView对象,并将“message”属性设置为“hello”。我们还设置了视图名称“hello”,这意味着DispatcherServlet将使用名为“hello”的视图来渲染响应。
我们还可以将模型数据添加到ModelAndView对象中,如下所示:
```
@RequestMapping(value = "/hello", method = RequestMethod.GET)
public ModelAndView hello() {
Map<String, Object> model = new HashMap<>();
model.put("message", "hello");
model.put("name", "John");
ModelAndView modelAndView = new ModelAndView("hello", model);
return modelAndView;
}
```
在上面的示例中,我们创建了一个包含两个模型属性的Map对象,并将其传递给ModelAndView构造函数。这些模型属性可以在视图中使用,如下所示:
```
<html>
<body>
<h1>${message} ${name}!</h1>
</body>
</html>
```
在上面的示例中,我们使用了EL表达式${message}和${name}来输出模型属性的值。
总之,使用ModelAndView可以很方便地返回数据和视图,它提供了一种解耦的方式来处理模型数据和视图。
阅读全文
相关推荐
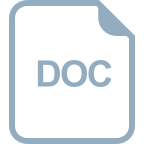
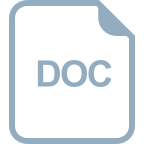
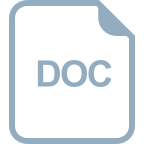




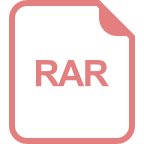
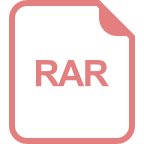
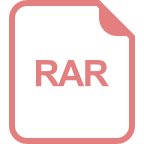
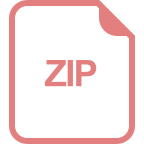
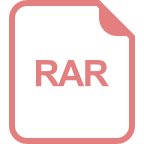
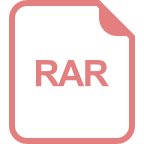
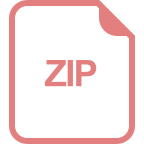
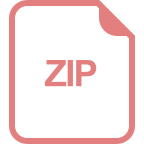

