巡回置换算法例题详解
时间: 2023-12-20 14:30:38 浏览: 139
巡回置换问题是指在一个完全图中,每个顶点之间都有一条边,求一条经过每个顶点一次且仅一次的回路,使得回路的总长度最小。这个问题是一个NP难问题,因此通常使用启发式算法来解决。其中一种启发式算法是遗传算法。
遗传算法是一种模拟自然进化过程的优化算法。在巡回置换问题中,可以将每个可能的回路看作一个个体,通过交叉、变异等操作来产生新的个体,并通过适应度函数来评估每个个体的优劣程度。经过多代进化,最终得到一个较优的回路。
以下是巡回置换问题的遗传算法的Python实现:
```python
import random
# 生成初始种群
def generate_population(city_num, pop_size):
population = []
for i in range(pop_size):
chromosome = list(range(city_num))
random.shuffle(chromosome)
population.append(chromosome)
return population
# 计算路径长度
def get_distance(city1, city2):
return ((city1[0] - city2[0]) ** 2 + (city1[1] - city2[1]) ** 2) ** 0.5
def get_path_length(path, cities):
length = 0
for i in range(len(path) - 1):
length += get_distance(cities[path[i]], cities[path[i+1]])
length += get_distance(cities[path[-1]], cities[path[0]])
return length
# 选择操作
def selection(population, cities):
fitness_list = [1 / get_path_length(chromosome, cities) for chromosome in population]
total_fitness = sum(fitness_list)
probability_list = [fitness / total_fitness for fitness in fitness_list]
selected_population = []
for i in range(len(population)):
selected_population.append(random.choices(population, probability_list)[0])
return selected_population
# 交叉操作
def crossover(parent1, parent2):
child = [-1] * len(parent1)
start = random.randint(0, len(parent1) - 1)
end = random.randint(0, len(parent1) - 1)
if start > end:
start, end = end, start
for i in range(start, end+1):
child[i] = parent1[i]
j = 0
for i in range(len(parent2)):
if child[j] == -1:
if parent2[i] not in child:
child[j] = parent2[i]
j += 1
else:
j += 1
return child
# 变异操作
def mutation(chromosome):
index1 = random.randint(0, len(chromosome) - 1)
index2 = random.randint(0, len(chromosome) - 1)
chromosome[index1], chromosome[index2] = chromosome[index2], chromosome[index1]
return chromosome
# 遗传算法主函数
def genetic_algorithm(city_list, pop_size, generation_num, crossover_rate, mutation_rate):
population = generate_population(len(city_list), pop_size)
for i in range(generation_num):
population = selection(population, city_list)
new_population = []
for j in range(pop_size):
parent1 = random.choice(population)
if random.random() < crossover_rate:
parent2 = random.choice(population)
child = crossover(parent1, parent2)
else:
child = parent1
if random.random() < mutation_rate:
child = mutation(child)
new_population.append(child)
population = new_population
best_path = min(population, key=lambda x: get_path_length(x, city_list))
best_length = get_path_length(best_path, city_list)
return best_path, best_length
# 测试
if __name__ == '__main__':
city_list = [(0, 0), (1, 1), (2, 2), (3, 3), (4, 4)]
best_path, best_length = genetic_algorithm(city_list, 100, 1000, 0.8, 0.1)
print('最优路径:', best_path)
print('最短路径长度:', best_length)
```
阅读全文
相关推荐










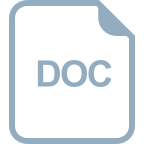






