c#获取cpu使用率
时间: 2023-09-13 22:12:36 浏览: 68
你可以使用 System.Diagnostics 命名空间下的 PerformanceCounter 类来获取 CPU 使用率。以下是一个示例代码:
```
using System.Diagnostics;
...
// 创建一个 PerformanceCounter 对象
PerformanceCounter cpuCounter = new PerformanceCounter("Processor", "% Processor Time", "_Total");
// 获取 CPU 使用率
float cpuUsage = cpuCounter.NextValue();
// 等待一段时间,然后再次获取 CPU 使用率
System.Threading.Thread.Sleep(1000);
cpuUsage = cpuCounter.NextValue();
```
在上面的代码中,我们首先创建了一个 PerformanceCounter 对象,指定了计数器的类别为 "Processor",计数器名称为 "% Processor Time",实例名称为 "_Total",这样就可以获取整个系统的 CPU 使用率。然后我们通过调用 NextValue 方法来获取 CPU 使用率的值。由于 CPU 使用率是实时变化的,因此我们在获取完一次值后等待一段时间再次获取,这样可以得到更准确的结果。
相关问题
c# 获取进程cpu使用率
你可以使用 System.Diagnostics 命名空间下的 Process 类来获取进程的 CPU 使用率。以下是一个示例代码:
```
using System.Diagnostics;
...
// 创建一个 Process 对象
Process process = Process.GetProcessById(processId);
// 创建一个 PerformanceCounter 对象
PerformanceCounter cpuCounter = new PerformanceCounter("Process", "% Processor Time", process.ProcessName, true);
// 获取进程 CPU 使用率
float cpuUsage = cpuCounter.NextValue();
// 等待一段时间,然后再次获取 CPU 使用率
System.Threading.Thread.Sleep(1000);
cpuUsage = cpuCounter.NextValue();
```
在上面的代码中,我们首先创建了一个 Process 对象,通过进程的 ID 获取到该进程的 Process 对象。然后我们创建了一个 PerformanceCounter 对象,指定了计数器的类别为 "Process",计数器名称为 "% Processor Time",实例名称为进程的名称,最后一个参数为 true 表示该计数器为多实例计数器,可以根据实例名称获取不同进程的 CPU 使用率。接着我们通过调用 NextValue 方法来获取进程 CPU 使用率的值,同样需要等待一段时间再次获取以得到更准确的结果。
C#获取CPU和内存使用率
你可以使用System.Diagnostics命名空间中的PerformanceCounter类来获取CPU和内存使用率。以下是使用C#获取CPU和内存使用率的示例代码:
获取CPU使用率:
```csharp
using System.Diagnostics;
PerformanceCounter cpuCounter;
cpuCounter = new PerformanceCounter("Processor", "% Processor Time", "_Total");
// 获取CPU使用率
float cpuUsage = cpuCounter.NextValue();
```
获取内存使用率:
```csharp
using System.Diagnostics;
PerformanceCounter memCounter;
memCounter = new PerformanceCounter("Memory", "Available MBytes");
// 获取可用内存
float availableMemory = memCounter.NextValue();
// 获取总内存
float totalMemory = new ComputerInfo().TotalPhysicalMemory / (1024 * 1024);
// 获取内存使用率
float memoryUsage = (totalMemory - availableMemory) / totalMemory * 100;
```
相关推荐
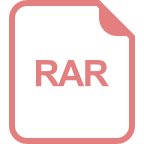












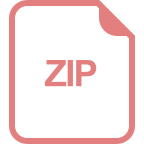