感知器python实现
时间: 2023-12-04 18:03:53 浏览: 65
感知器是一种二分类的线性分类器,它的基本思想是利用输入信息的线性组合,对输入进行二分类。感知器的实现可以使用Python语言来完成。
首先,需要定义感知器的类。在这个类中,需要定义感知器的初始化方法,训练方法和预测方法。初始化方法需要定义感知器的权重和偏置,训练方法需要根据训练数据来更新感知器的权重和偏置,预测方法需要根据输入数据和感知器的权重和偏置来判断输入数据是属于哪一类。
代码如下:
```python
import numpy as np
class Perceptron:
def __init__(self, learning_rate=0.1, epochs=1000):
self.learning_rate = learning_rate
self.epochs = epochs
self.weights = None
self.bias = None
def fit(self, X, y):
n_samples, n_features = X.shape
self.weights = np.zeros(n_features)
self.bias = 0
y_ = np.where(y <= 0, -1, 1)
for _ in range(self.epochs):
for idx, x_i in enumerate(X):
linear_output = np.dot(x_i, self.weights) + self.bias
y_predicted = np.where(linear_output <= 0, -1, 1)
update = self.learning_rate * (y_[idx] - y_predicted)
self.weights += update * x_i
self.bias += update
def predict(self, X):
linear_output = np.dot(X, self.weights) + self.bias
y_predicted = np.where(linear_output <= 0, -1, 1)
return y_predicted
```
以上代码中,Perceptron类的初始化方法中包含学习率和迭代次数等参数。在训练方法中,首先将标签y的值转换为-1和1,然后根据迭代次数和输入数据更新感知器的权重和偏置。在预测方法中,通过感知器的权重和偏置进行线性组合,然后根据线性输出的值判断输入数据是属于哪一类。
下面是一个简单的例子,用于说明如何使用感知器进行分类:
```python
X = np.array([[2, 3], [1, 1.5], [3, 4], [4, 4], [1.5, 2], [2.5, 3], [2, 2], [4.5, 5]])
y = np.array([1, -1, 1, 1, -1, 1, -1, 1])
perceptron = Perceptron()
perceptron.fit(X, y)
X_test = np.array([[1, 1], [3, 3.5], [2.5, 2]])
y_pred = perceptron.predict(X_test)
print(y_pred)
# Output: [-1 1 -1]
```
以上代码中,我们使用了一组二维数据和标签,然后通过Perceptron类进行训练和预测,最终得到了数据的分类结果。
阅读全文
相关推荐
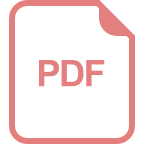

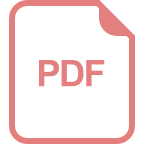
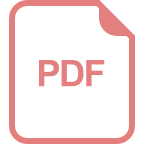
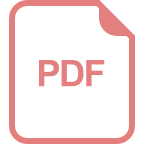
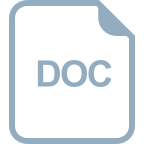
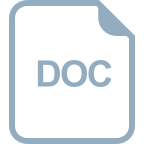





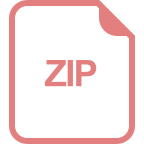