c++ sort 用法
时间: 2024-09-05 16:04:49 浏览: 33
`sort` 是 C++ 标准库中的一个函数,用于对数组或容器中的元素进行排序。`sort` 函数定义在 `<algorithm>` 头文件中,其基本用法是使用默认的比较函数(即升序排列),也可以通过自定义比较函数来实现其他类型的排序。
以下是 `sort` 函数的基本用法:
1. 升序排列,使用默认比较函数:
```cpp
#include <algorithm>
#include <vector>
int main() {
std::vector<int> vec = {3, 1, 4, 1, 5, 9, 2, 6};
std::sort(vec.begin(), vec.end()); // vec 现在是 {1, 1, 2, 3, 4, 5, 6, 9}
// ...
}
```
2. 降序排列,使用自定义比较函数:
```cpp
#include <algorithm>
#include <vector>
#include <functional> // for std::greater
int main() {
std::vector<int> vec = {3, 1, 4, 1, 5, 9, 2, 6};
std::sort(vec.begin(), vec.end(), std::greater<int>()); // vec 现在是 {9, 6, 5, 4, 3, 2, 1, 1}
// ...
}
```
3. 对自定义类型的数组或容器进行排序,需要定义比较规则:
```cpp
#include <algorithm>
#include <vector>
#include <string>
struct MyStruct {
std::string name;
int age;
};
bool compareMyStruct(const MyStruct &a, const MyStruct &b) {
return a.age < b.age; // 升序排列年龄
}
int main() {
std::vector<MyStruct> structs = {
{"Alice", 30},
{"Bob", 25},
{"Charlie", 35}
};
std::sort(structs.begin(), structs.end(), compareMyStruct); // 根据年龄升序排列
// ...
}
```
相关推荐
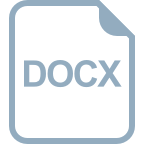
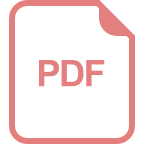
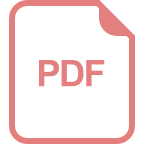














