C#读取文本数据到字符串
时间: 2023-09-09 18:14:32 浏览: 131
你可以使用C#的StreamReader类来读取文本数据到字符串中。以下是一个简单的示例代码:
```csharp
using System;
using System.IO;
class Program {
static void Main(string[] args) {
string filePath = "example.txt"; // 文本文件路径
string textData = "";
try {
using (StreamReader sr = new StreamReader(filePath)) {
textData = sr.ReadToEnd(); // 读取整个文本文件内容到字符串中
}
}
catch (Exception e) {
Console.WriteLine("读取文件失败: " + e.Message);
}
Console.WriteLine("文本数据: " + textData);
}
}
```
在上面的示例代码中,我们使用StreamReader类来打开指定的文本文件,然后使用ReadToEnd()方法将整个文本文件内容读取到字符串textData中。最后,我们输出textData字符串中的文本数据。
相关问题
C#字符串读取txt
你可以使用C#中的System.IO命名空间中的StreamReader类来读取文本文件中的字符串。以下是一个示例代码,可以读取指定路径下的文本文件,并将内容输出到控制台:
```
using System.IO;
string filePath = "your_file_path.txt";
// 创建一个StreamReader对象来读取文件
StreamReader reader = new StreamReader(filePath);
// 读取文件中的所有内容并输出到控制台
Console.WriteLine(reader.ReadToEnd());
// 关闭StreamReader对象
reader.Close();
```
注意,在读取文本文件时,需要确保文件路径正确,并且需要适当地处理异常情况。
C#字符串读取txt并且转ascll
你可以使用C#中的System.IO命名空间中的StreamReader类来读取文本文件中的字符串,并使用System.Text中的Encoding.ASCII.GetBytes()方法将读取的字符串转换成ASCII码。以下是一个示例代码,可以读取指定路径下的文本文件,并将内容转换成ASCII码输出到控制台:
```
using System;
using System.IO;
using System.Text;
string filePath = "your_file_path.txt";
// 创建一个StreamReader对象来读取文件
StreamReader reader = new StreamReader(filePath);
// 读取文件中的所有内容
string content = reader.ReadToEnd();
// 关闭StreamReader对象
reader.Close();
// 将读取的字符串转换成ASCII码
byte[] asciiBytes = Encoding.ASCII.GetBytes(content);
// 输出转换后的ASCII码到控制台
foreach (byte b in asciiBytes)
{
Console.Write(b + " ");
}
```
注意,在转换字符串为ASCII码时,也需要确保文件路径正确,并且需要适当地处理异常情况。
相关推荐
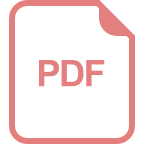












