python实现GA-xgboost
时间: 2023-10-24 07:06:42 浏览: 85
GA-XGBoost是一种使用遗传算法优化XGBoost模型的方法。在这种方法中,使用遗传算法来搜索XGBoost模型的最佳超参数组合。这种方法的实现需要使用Python编程语言。具体来说,需要使用Python中的遗传算法库和XGBoost库。在实现过程中,需要进行初始化、适应度评估、选择、交叉和变异等步骤。其中,适应度评估是指使用XGBoost模型对训练数据进行拟合,并计算模型的性能指标,例如准确率、召回率和F1分数等。通过遗传算法的迭代过程,可以找到最佳的超参数组合,从而得到最优的XGBoost模型。
下面是一个简单的Python代码示例,用于初始化GA-XGBoost算法:
```
import numpy as np
import xgboost as xgb
from deap import base, creator, tools
# 初始化遗传算法参数
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
toolbox = base.Toolbox()
# 定义参数范围
param_space = {
'learning_rate': np.arange(0.01, 0.2, 0.01),
'n_estimators': range(50, 500, 50),
'max_depth': range(3, 10),
'min_child_weight': range(1, 6),
'subsample': np.arange(0.5, 1.0, 0.1),
'colsample_bytree': np.arange(0.5, 1.0, 0.1),
'gamma': np.arange(0, 1.0, 0.1)
}
# 定义遗传算法操作
toolbox.register("attr_float", np.random.uniform, 0, 1)
toolbox.register("individual", tools.initCycle, creator.Individual,
(toolbox.attr_float,)*len(param_space), n=1)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 定义适应度评估函数
def evaluate(individual):
params = {k: param_space[k][int(v*len(param_space[k]))] for k, v in zip(param_space.keys(), individual)}
model = xgb.XGBClassifier(**params)
model.fit(X_train, y_train)
score = model.score(X_test, y_test)
return score,
toolbox.register("evaluate", evaluate)
```
相关推荐
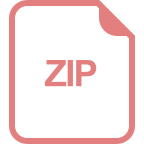
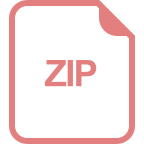
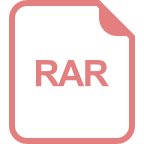













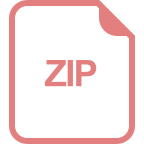
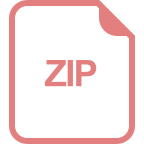