编写生产者类、消费者类及共享资源类,然后用主类来测试同步问题。产品在生产和消费的过程中必须保证产品数据更新的同步,也就是说当生产一个产品之后,生产者和消费者的产品(Shares)的数量都要增加1,这样才能保证数据的一致性。在共享资源类Shares的get()和put()方法中添加了synchronized关键字,并且通过wait()方法和notify()方法,实现了线程的同步。
时间: 2024-03-01 21:54:05 浏览: 21
好的,以下是一个简单的Java生产者-消费者模型示例代码:
共享资源类Shares:
```java
public class Shares {
private int count = 0;
private final int MAX_COUNT = 10;
public synchronized void put() {
while (count >= MAX_COUNT) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
count++;
System.out.println("生产者生产了一个产品,当前产品数量为:" + count);
notifyAll();
}
public synchronized void get() {
while (count <= 0) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
count--;
System.out.println("消费者消费了一个产品,当前产品数量为:" + count);
notifyAll();
}
}
```
生产者类Producer:
```java
public class Producer implements Runnable {
private Shares shares;
public Producer(Shares shares) {
this.shares = shares;
}
@Override
public void run() {
while (true) {
shares.put();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
消费者类Consumer:
```java
public class Consumer implements Runnable {
private Shares shares;
public Consumer(Shares shares) {
this.shares = shares;
}
@Override
public void run() {
while (true) {
shares.get();
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
主类Main:
```java
public class Main {
public static void main(String[] args) {
Shares shares = new Shares();
Producer producer = new Producer(shares);
Consumer consumer = new Consumer(shares);
Thread thread1 = new Thread(producer);
Thread thread2 = new Thread(consumer);
thread1.start();
thread2.start();
}
}
```
该代码创建了一个共享资源类Shares,其中包含put()方法和get()方法,用于生产和消费产品。在put()方法和get()方法中使用synchronized关键字实现了线程同步,并通过wait()方法和notify()方法来实现线程等待和唤醒。同时,主类Main创建了一个生产者实例和一个消费者实例,并分别启动了两个线程来执行生产和消费操作。运行该程序,可以看到生产者和消费者之间的同步效果。
相关推荐
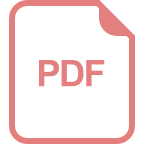
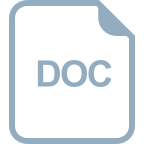
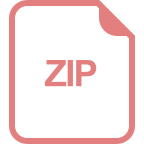
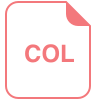
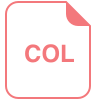
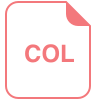











