这道题目的意思是让你编写一个函数模板,使用冒泡排序将数组内容由小到大排列并打印出来,同时写出调用此函数模板的完整程序,使得函数调用时,数组的类型可以是整型也可以是双精度型
时间: 2023-06-20 08:10:21 浏览: 111
好的,我来为你编写这个函数模板和调用程序。
函数模板:
```cpp
template <typename T>
void bubbleSort(T arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
T temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
```
完整程序:
```cpp
#include <iostream>
using namespace std;
template <typename T>
void bubbleSort(T arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
T temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
int arr1[] = {3, 5, 2, 1, 4};
double arr2[] = {1.3, 2.1, 0.5, 3.6, 2.9};
int n1 = sizeof(arr1) / sizeof(arr1[0]);
int n2 = sizeof(arr2) / sizeof(arr2[0]);
cout << "Before sorting:" << endl;
for (int i = 0; i < n1; i++) {
cout << arr1[i] << " ";
}
cout << endl;
cout << "After sorting:" << endl;
bubbleSort(arr1, n1);
for (int i = 0; i < n1; i++) {
cout << arr1[i] << " ";
}
cout << endl;
cout << "Before sorting:" << endl;
for (int i = 0; i < n2; i++) {
cout << arr2[i] << " ";
}
cout << endl;
cout << "After sorting:" << endl;
bubbleSort(arr2, n2);
for (int i = 0; i < n2; i++) {
cout << arr2[i] << " ";
}
cout << endl;
return 0;
}
```
这个程序中,我们首先定义了一个函数模板 `bubbleSort`,接收一个类型为 `T` 的数组和数组的长度 `n`。
在 `main` 函数中,我们定义了两个数组 `arr1` 和 `arr2`,并获取它们的长度。我们使用 `cout` 输出数组排序前的元素,然后调用 `bubbleSort` 函数对数组进行排序,并再次使用 `cout` 输出排序后的元素。
注意,在程序中要加上 `using namespace std;`,以使用 `cout` 和 `endl` 等标准库函数。
阅读全文
相关推荐
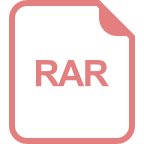
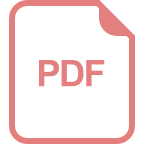
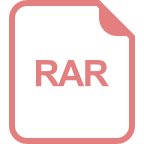
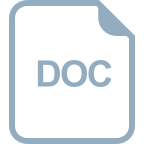
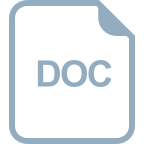
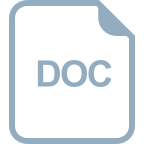
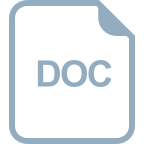
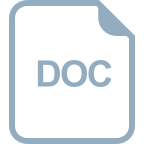
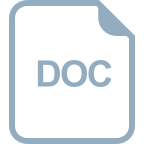
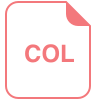
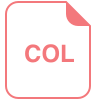
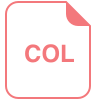
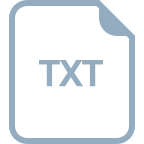
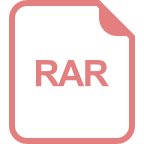