struct chexinxi *duqu() { struct chexinxi *head2= NULL; struct chexinxi *temp = NULL; char chexinghao[20], chepaihao[20]; FILE *fp = fopen("车辆信息.txt", "r"); if (fp == NULL) { printf("\t\t\t\t打开文件失败!文件名: 车辆信息.txt\n"); return NULL; } else { while (fscanf(fp, "%s %s", chexinghao, chepaihao) != EOF) { struct chexinxi *p = (struct chexinxi *)malloc(sizeof(struct chexinxi)); if (p == NULL) { printf("分配内存失败!\n"); } strcpy(p->chexinghao, chexinghao); strcpy(p->chepaihao, chepaihao); if (head2 == NULL) { head2 = temp = p; } else { temp->next = p; temp = p; } } fclose(fp); if (temp != NULL) { temp->next = NULL; } return head2; } }为什么错了
时间: 2024-02-15 13:29:07 浏览: 63
我看不出这段代码有什么明显的错误。但是,如果你遇到了问题,可能是由于以下几个原因:
1. 文件名或路径不正确:请确保文件名和路径与实际情况相符。
2. 内存分配失败:如果内存分配失败,则可能是由于没有足够的可用内存。请确保你的系统中有足够的可用内存,并尝试使用内存分配失败时返回的错误代码来找出问题所在。
3. 文件格式不正确:如果文件中的数据格式不正确,则可能会导致读取错误。请确保文件中的数据格式与代码中使用的格式相同。
如果你能提供更多详细的信息,我可能会更好地帮助你解决问题。
相关问题
struct chexinxi *duqu() { struct chexinxi *head2=NULL; struct chexinxi *p,*pr; char chexinghao[20], chepaihao[20]; FILE *fp = fopen("车辆信息.txt", "r"); if(fp==NULL) { printf("\t\t\t\t打开文件失败!\n"); } else { while (fscanf(fp, "%s %s", chexinghao, chepaihao) != EOF) { p=(struct chexinxi*)malloc(sizeof(struct chexinxi)); strcpy(p->chexinghao, chexinghao); strcpy(p->chepaihao, chepaihao); if (head2 == NULL) { pr=head2 = p; } else { pr->next=p; pr=p; } } fclose(fp); pr->next=NULL; } return head2; }优化
下面是对这段代码的优化:
1. 添加错误处理。在打开文件失败时,应该输出更多的错误信息,例如文件名和错误码等,方便用户进行问题定位。
2. 简化链表构建过程。可以使用一个临时指针变量 temp,将其始终指向链表的尾节点,将新节点插入到链表的尾部,可以避免大量的 if/else 语句。
3. 检查 malloc 分配内存是否成功。如果分配内存失败,应该进行相应的错误处理。
优化后的代码如下所示:
```c
struct chexinxi *duqu() {
struct chexinxi *head = NULL;
struct chexinxi *temp = NULL;
char chexinghao[20], chepaihao[20];
FILE *fp = fopen("车辆信息.txt", "r");
if (fp == NULL) {
printf("打开文件失败!文件名:%s,错误码:%d\n", "车辆信息.txt", errno);
} else {
while (fscanf(fp, "%s %s", chexinghao, chepaihao) != EOF) {
struct chexinxi *p = malloc(sizeof(struct chexinxi));
if (p == NULL) {
printf("分配内存失败!\n");
exit(1);
}
strcpy(p->chexinghao, chexinghao);
strcpy(p->chepaihao, chepaihao);
if (head == NULL) {
head = temp = p;
} else {
temp->next = p;
temp = p;
}
}
fclose(fp);
if (temp != NULL) {
temp->next = NULL;
}
}
return head;
}
```
通过以上优化,代码的可读性和健壮性都得到了提高。
struct chexinxi *duqu() { struct chexinxi *p,*head2,*pr; FILE *fp = fopen("车辆信息.txt", "r"); if(fp==NULL) { printf("\t\t\t\t打开文件失败!\n"); } else { while (fscanf(fp, "%s %s", chexinghao, chepaihao) != EOF) { struct chexinxi *p = (struct chexinxi*)malloc(sizeof(struct chexinxi)); strcpy(p->chexinghao, chexinghao); strcpy(p->chepaihao, chepaihao); p->next = NULL; if (head2 == NULL) { head2 = p; } else { struct chexinxi *pr = head; while (pr->next != NULL) { pr=pr->next; } pr->next = p; } } fclose(fp); } return head2; }为什么错了
这段代码中存在几个问题:
1. 在函数中,`head2`没有被初始化,而在第一次使用时直接判断是否为NULL,这会导致未定义的行为。应该在函数开始时将其初始化为NULL。
2. 在while循环中,使用了一个新的指针变量`p`,但是在内部并没有将其赋值给外部的`head2`,这意味着即使在循环中成功地创建了新的节点,也不会将其添加到链表中。
3. 在`else`分支中,将`pr`初始化为`head`,但是`head`并没有在函数中声明或初始化,这也会导致未定义的行为。
4. 在`else`分支中,将新节点添加到链表末尾的代码有误,应该先将`pr`移动到链表的末尾,然后再将新节点添加到末尾。
针对以上问题,可以将代码修改为以下形式:
```
struct chexinxi *duqu() {
struct chexinxi *head2 = NULL;
struct chexinxi *p, *pr;
char chexinghao[20], chepaihao[20];
FILE *fp = fopen("车辆信息.txt", "r");
if (fp == NULL) {
printf("\t\t\t\t打开文件失败!\n");
} else {
while (fscanf(fp, "%s %s", chexinghao, chepaihao) != EOF) {
p = (struct chexinxi*)malloc(sizeof(struct chexinxi));
strcpy(p->chexinghao, chexinghao);
strcpy(p->chepaihao, chepaihao);
p->next = NULL;
if (head2 == NULL) {
head2 = p;
} else {
pr = head2;
while (pr->next != NULL) {
pr = pr->next;
}
pr->next = p;
}
}
fclose(fp);
}
return head2;
}
```
这样应该可以解决代码中的问题。
阅读全文
相关推荐
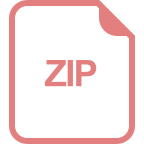
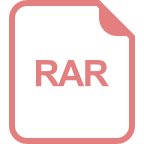







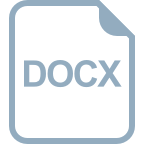
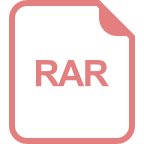