java的快速排序怎么写?
时间: 2023-09-15 18:05:10 浏览: 71
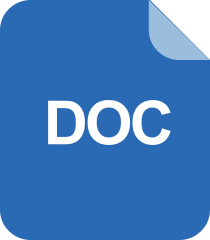
快速排序算法 java.doc
### 回答1:
Java 快速排序的代码如下:
```
import java.util.Arrays;
public class QuickSort {
public static void main(String[] args) {
int[] nums = {3, 9, 1, 4, 7};
quickSort(nums, 0, nums.length - 1);
System.out.println(Arrays.toString(nums));
}
public static void quickSort(int[] nums, int low, int high) {
if (low < high) {
int pivotIndex = partition(nums, low, high);
quickSort(nums, low, pivotIndex - 1);
quickSort(nums, pivotIndex + 1, high);
}
}
public static int partition(int[] nums, int low, int high) {
int pivot = nums[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (nums[j] <= pivot) {
i++;
swap(nums, i, j);
}
}
swap(nums, i + 1, high);
return i + 1;
}
public static void swap(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
}
```
这段代码使用了递归的方式实现了快速排序算法。
详细说明:
- `quickSort` 函数:如果 `low` 小于 `high`,则调用 `partition` 函数对数组进行划分,然后递归地对划分后的左半边和右半边分别进行排序。
- `partition` 函数:选取最后一个数作为枢轴,遍历数组,将小于枢轴的数移到左边,最后再将枢轴换到合适的位置。
- `swap` 函数:交换数组中两个元素的位置。
### 回答2:
快速排序是一种常见且高效的排序算法,也是Java中常用的排序算法之一。快速排序的基本思想是通过一趟排序将待排序序列分割成独立的两部分,其中一部分的元素都比另一部分小,然后再对这两部分分别进行排序,以达到整个序列有序的目的。
下面是Java实现快速排序的代码:
```
public class QuickSort {
public static void main(String[] args) {
int[] arr = {5, 2, 6, 1, 3, 9, 4, 8, 7};
quickSort(arr, 0, arr.length - 1);
for (int num : arr) {
System.out.print(num + " ");
}
}
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIdx = partition(arr, low, high);
quickSort(arr, low, pivotIdx - 1);
quickSort(arr, pivotIdx + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low]; // 选择第一个元素作为基准值
while (low < high) {
while (low < high && arr[high] >= pivot) {
high--;
}
arr[low] = arr[high];
while (low < high && arr[low] <= pivot) {
low++;
}
arr[high] = arr[low];
}
arr[low] = pivot;
return low;
}
}
```
以上代码实现了使用快速排序算法对一个整数数组进行排序。通过`quickSort`方法实现递归调用,`partition`方法实现了快速排序的核心逻辑。其中通过选择第一个元素作为基准值,将比基准值大的元素移到右边,将比基准值小的元素移到左边。再递归对左右两部分进行排序,最终得到有序序列。
以上就是用于Java的快速排序的实现方法。
### 回答3:
快速排序是一种常用的排序算法,其主要思想是通过选择一个基准元素,将数组分成两部分,一部分小于基准元素,一部分大于基准元素,然后对两部分分别进行递归排序,最终实现整个数组的有序排列。
Java的快速排序可以使用递归的方式实现,代码如下:
```java
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high); // 划分数组
quickSort(arr, low, pivotIndex - 1); // 对左子数组进行快速排序
quickSort(arr, pivotIndex + 1, high); // 对右子数组进行快速排序
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low]; // 选择第一个元素作为基准元素
int left = low;
int right = high;
while (left < right) {
// 从右往左找到第一个小于基准元素的数
while (left < right && arr[right] >= pivot) {
right--;
}
// 从左往右找到第一个大于基准元素的数
while (left < right && arr[left] <= pivot) {
left++;
}
// 交换两个数的位置
int temp = arr[left];
arr[left] = arr[right];
arr[right] = temp;
}
// 将基准元素放到最终位置(left == right)
arr[low] = arr[left];
arr[left] = pivot;
return left;
}
public static void main(String[] args) {
int[] arr = {10, 7, 8, 9, 1, 5};
int n = arr.length;
quickSort(arr, 0, n - 1);
System.out.println("排序后的数组:");
for (int i : arr) {
System.out.print(i + " ");
}
}
}
```
以上就是使用Java实现快速排序的代码。在`quickSort`方法中,我们首先选择一个基准元素,并根据基准元素的位置进行划分。然后对左右子数组进行递归调用,直到每个子数组都被排序。最后,通过不断交换元素的位置,将基准元素放到最终位置上。最终输出排序后的数组。
阅读全文
相关推荐
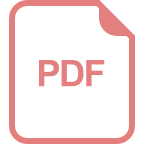
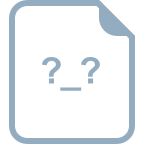
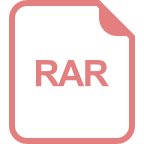
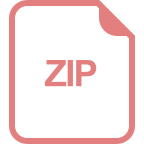
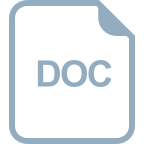
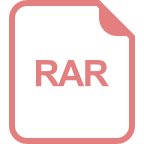
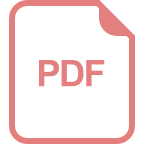
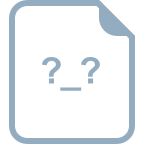
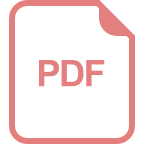