c语言文件读写操作代码
时间: 2024-01-18 14:03:19 浏览: 44
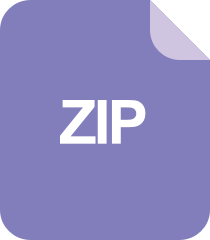
C语言文件的读写操作代码
以下是 C 语言中文件读写操作的常用代码示例:
1. 文件读取操作
```c
#include <stdio.h>
int main() {
FILE *fp;
char buffer[255];
fp = fopen("example.txt", "r");
if (fp == NULL) {
printf("File does not exist!");
return 0;
}
// 从文件中读取一行数据
fgets(buffer, 255, fp);
printf("%s", buffer);
fclose(fp);
return 0;
}
```
2. 文件写入操作
```c
#include <stdio.h>
int main() {
FILE *fp;
fp = fopen("example.txt", "w");
if (fp == NULL) {
printf("File does not exist!");
return 0;
}
// 写入数据到文件中
fprintf(fp, "This is an example file.\n");
fputs("This is another line.", fp);
fclose(fp);
return 0;
}
```
3. 二进制文件读取操作
```c
#include <stdio.h>
int main() {
FILE *fp;
int n;
float x;
fp = fopen("example.bin", "rb");
if (fp == NULL) {
printf("File does not exist!");
return 0;
}
// 从二进制文件中读取数据
fread(&n, sizeof(int), 1, fp);
fread(&x, sizeof(float), 1, fp);
printf("n = %d, x = %f", n, x);
fclose(fp);
return 0;
}
```
4. 二进制文件写入操作
```c
#include <stdio.h>
int main() {
FILE *fp;
int n = 10;
float x = 3.14;
fp = fopen("example.bin", "wb");
if (fp == NULL) {
printf("File does not exist!");
return 0;
}
// 将数据写入二进制文件中
fwrite(&n, sizeof(int), 1, fp);
fwrite(&x, sizeof(float), 1, fp);
fclose(fp);
return 0;
}
```
阅读全文
相关推荐
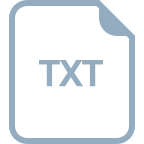
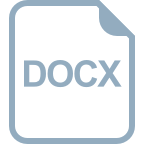
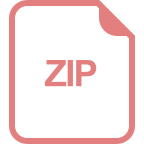
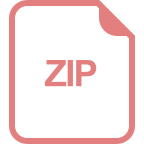
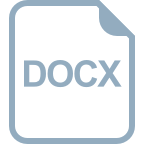
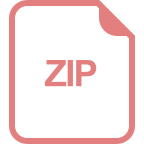
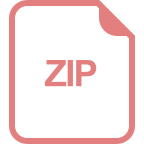