pyqt5 graphicsView 加载显示图片,在图片上鼠标框选红色矩形并返回矩形在图片上的坐标
时间: 2023-12-22 17:05:44 浏览: 60
可以通过继承 QGraphicsView 和 QGraphicsScene 类,实现在图片上框选矩形并返回矩形坐标的功能。以下是示例代码:
```python
from PyQt5.QtWidgets import QGraphicsView, QGraphicsScene
from PyQt5.QtCore import Qt, QRectF, QPointF
from PyQt5.QtGui import QPixmap, QPen, QColor
class ImageViewer(QGraphicsView):
def __init__(self):
super().__init__()
self.setScene(QGraphicsScene(self))
self.setDragMode(QGraphicsView.ScrollHandDrag)
self.setRenderHint(QPainter.Antialiasing)
self.setRenderHint(QPainter.SmoothPixmapTransform)
self.setTransformationAnchor(QGraphicsView.AnchorUnderMouse)
self.setHorizontalScrollBarPolicy(Qt.ScrollBarAlwaysOff)
self.setVerticalScrollBarPolicy(Qt.ScrollBarAlwaysOff)
self.setFixedSize(800, 600)
self._start = QPointF()
self._rect_item = None
def loadImage(self, img_path):
pixmap = QPixmap(img_path)
self.setSceneRect(pixmap.rect())
self.scene().addPixmap(pixmap)
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
self._start = event.pos()
pen = QPen(QColor(255, 0, 0))
self._rect_item = self.scene().addRect(QRectF(), pen)
self._rect_item.setZValue(1)
def mouseMoveEvent(self, event):
if event.buttons() == Qt.LeftButton:
rect = QRectF(self._start, event.pos()).normalized()
self._rect_item.setRect(rect)
def mouseReleaseEvent(self, event):
if event.button() == Qt.LeftButton:
rect = self._rect_item.rect()
img_rect = self.sceneRect()
x = rect.x() / img_rect.width()
y = rect.y() / img_rect.height()
w = rect.width() / img_rect.width()
h = rect.height() / img_rect.height()
print("Rect coordinates:", x, y, w, h)
```
在以上代码中,loadImage() 方法可以将图片加载到场景中。mousePressEvent()、mouseMoveEvent() 和 mouseReleaseEvent() 方法可以实现鼠标在图片上框选矩形并记录矩形坐标的功能。其中,x、y、w、h 分别为矩形的左上角坐标和宽高的比例值,通过这些值可以计算出矩形在图片上的真实坐标。
阅读全文
相关推荐
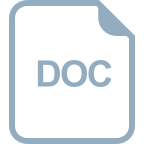
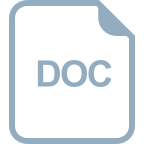
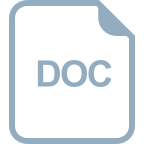








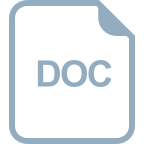
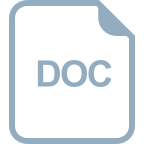
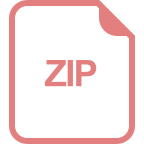
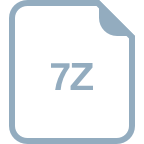
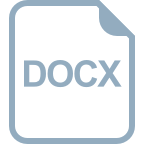