用Java连接数据库,编程实现往表中插入自己和张三的名字和学号,再查询表中记录,并把表记录打印在控制台上。
时间: 2024-02-28 22:56:47 浏览: 16
下面是Java代码实现连接数据库,往表中插入自己和张三的名字和学号,再查询表中记录,并把表记录打印在控制台上的示例:
```java
import java.sql.*;
public class DatabaseExample {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 加载JDBC驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 建立数据库连接
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
// 往表中插入自己和张三的名字和学号
stmt = conn.createStatement();
stmt.executeUpdate("INSERT INTO student (name, id) VALUES ('Me', '001'), ('Zhang San', '002')");
// 查询表中记录并打印在控制台上
rs = stmt.executeQuery("SELECT * FROM student");
while (rs.next()) {
String name = rs.getString("name");
String id = rs.getString("id");
System.out.println("Name: " + name + ", ID: " + id);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (rs != null)
rs.close();
if (stmt != null)
stmt.close();
if (conn != null)
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
这段代码首先加载了JDBC驱动程序,然后建立了一个MySQL数据库连接。接着使用Statement对象执行SQL语句,往表中插入自己和张三的名字和学号。然后再次使用Statement对象执行SQL查询语句,将查询结果保存在ResultSet对象中,并使用while循环遍历ResultSet对象,将每个记录的姓名和学号打印在控制台上。最后关闭了ResultSet、Statement和Connection对象。
相关推荐
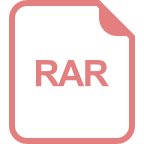
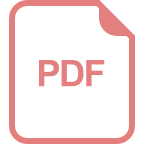
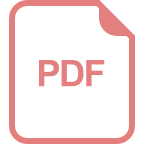














