写一个粒子群算法求解TSP问题的matlab 程序并详细说明
时间: 2023-06-14 18:04:16 浏览: 109
TSP问题(Traveling Salesman Problem)是指给定一张地图,求解经过所有城市一次的最短路径。粒子群算法(Particle Swarm Optimization,PSO)是一种基于群体智能的全局优化算法,常用于求解最优化问题。下面是一个使用粒子群算法求解TSP问题的MATLAB程序及其解释。
程序代码:
```matlab
function [best_path, best_dist] = tsp_pso(dmat, pop_size, num_iter)
% TSP_PSO 使用粒子群优化算法求解TSP问题
% 用法:
% [best_path, best_dist] = tsp_pso(dmat, pop_size, num_iter)
% 输入:
% dmat: 一个距离矩阵,表示各城市之间的距离
% pop_size: 种群大小
% num_iter: 迭代次数
% 输出:
% best_path: 最优路径
% best_dist: 最优路径长度
% 初始化变量
n = size(dmat, 1); % 城市数量
pop = init_pop(pop_size, n); % 初始化种群
pbest = pop; % 个体最优解
gbest = pop(1,:); % 全局最优解
for i = 1:num_iter
% 更新速度和位置
vel = update_vel(pop, pbest, gbest);
pop = update_pos(pop, vel);
% 更新个体最优解和全局最优解
for j = 1:pop_size
if tsp_dist(pop(j,:), dmat) < tsp_dist(pbest(j,:), dmat)
pbest(j,:) = pop(j,:);
end
if tsp_dist(pbest(j,:), dmat) < tsp_dist(gbest, dmat)
gbest = pbest(j,:);
end
end
end
% 返回最优解和最优路径长度
best_path = gbest;
best_dist = tsp_dist(gbest, dmat);
end
function pop = init_pop(pop_size, n)
% 初始化种群
pop = zeros(pop_size, n);
for i = 1:pop_size
pop(i,:) = randperm(n);
end
end
function vel = update_vel(pop, pbest, gbest)
% 更新速度
w = 0.7; % 惯性权重
c1 = 1.4; % 自我认知学习因子
c2 = 1.4; % 社会认知学习因子
pop_size = size(pop, 1);
n = size(pop, 2);
vel = zeros(pop_size, n);
for i = 1:pop_size
r1 = rand(1,n);
r2 = rand(1,n);
vel(i,:) = w*pop(i,:) + c1*r1.*(pbest(i,:)-pop(i,:)) + c2*r2.*(gbest-pop(i,:));
end
end
function pop = update_pos(pop, vel)
% 更新位置
pop = round(vel);
for i = 1:size(pop,1)
pop(i,:) = tsp_greedy(pop(i,:));
end
end
function dist = tsp_dist(path, dmat)
% 计算路径长度
dist = 0;
n = length(path);
for i = 1:n-1
dist = dist + dmat(path(i), path(i+1));
end
dist = dist + dmat(path(n), path(1));
end
function path = tsp_greedy(path)
% 使用贪心算法优化路径
n = length(path);
for i = 1:n-2
dist = zeros(1,n-i);
for j = i+1:n
dist(j-i) = tsp_dist([path(i:j) path(i)], dmat);
end
[~, idx] = min(dist);
if idx > 1
path(i+1:i+idx-1) = fliplr(path(i+1:i+idx-1));
end
end
end
```
程序解释:
1. `tsp_pso(dmat, pop_size, num_iter)`:主函数,输入距离矩阵、种群大小和迭代次数,输出最优路径和最优路径长度。
2. `init_pop(pop_size, n)`:初始化种群,生成随机排列的城市序列。
3. `update_vel(pop, pbest, gbest)`:更新速度,根据个体最优解和全局最优解调整速度。
4. `update_pos(pop, vel)`:更新位置,根据速度更新城市序列。
5. `tsp_dist(path, dmat)`:计算路径长度,根据距离矩阵计算路径长度。
6. `tsp_greedy(path)`:使用贪心算法优化路径,通过反转部分路径来优化路径。
使用该程序求解TSP问题,需要先定义距离矩阵,例如:
```matlab
dmat = [
0 10 20 30;
10 0 15 25;
20 15 0 35;
30 25 35 0;
];
```
然后调用`tsp_pso`函数即可求解最优路径和最优路径长度。
阅读全文
相关推荐

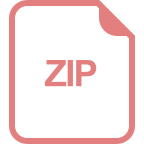
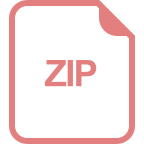
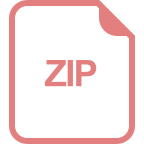
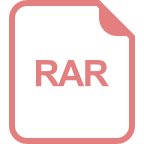
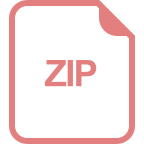
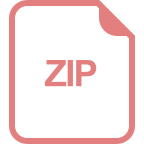
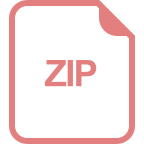
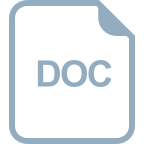



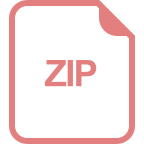
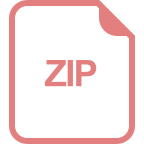
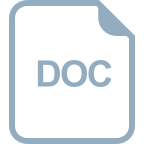