题目描述 给定一个年份,判断其是平年还是闰年。(提示:如果年份是400的倍数,或者是4的倍数但不是100的倍数,那么称这个年份为闰年) 输入描述 一个正整数nn(1900≤n≤99991900≤n≤9999)。 输出描述 如果是闰年,那么输出YES,否则输出NO。用C语言
时间: 2024-02-01 08:15:03 浏览: 33
以下是C语言实现:
```c
#include <stdio.h>
int main() {
int year;
scanf("%d", &year);
if (year % 400 == 0 || (year % 4 == 0 && year % 100 != 0)) {
printf("YES\n");
} else {
printf("NO\n");
}
return 0;
}
```
代码解释:
1. 首先,通过 `scanf` 函数输入一个正整数 `year`。
2. 然后,使用 if 语句判断是否是闰年,如果是,输出 "YES";否则,输出 "NO"。
3. 闰年的判断条件是:能被400整除或者能被4整除但不能被100整除。
相关问题
题目描述 给定一个年份,判断其是平年还是闰年。(提示:如果年份是400的倍数,或者是4的倍数但不是100的倍数,那么称这个年份为闰年) 输入描述 一个正整数nn(1900≤n≤99991900≤n≤9999)。 输出描述 如果是闰年,那么输出YES,否则输出NO 用c语言
以下是 C 语言代码实现:
```c
#include <stdio.h>
int main() {
int year;
scanf("%d", &year);
if (year % 400 == 0 || (year % 4 == 0 && year % 100 != 0)) {
printf("YES\n");
} else {
printf("NO\n");
}
return 0;
}
```
解释一下代码的逻辑:
1. 通过 `scanf` 函数读入一个整数 `year`,表示年份;
2. 根据题目描述,判断是否为闰年,如果是,则输出 `YES`,否则输出 `NO`;
3. 如果年份能被 400 整除,或者能被 4 整除但不能被 100 整除,则为闰年。
注意事项:
1. 如果年份是 1900 年,那么输出 `NO`,因为 1900 年能被 100 整除但不能被 400 整除;
2. 如果年份是 2000 年,那么输出 `YES`,因为 2000 年能被 400 整除。
输入年份和月份,输出该月的天数。闰年的2月是29天,平年28天,需增加对闰年的判断。 当以下情况之一满足时,这一年是闰年: 1.年份是4的倍数而不是100的倍数; 年份是400的倍数。 其他的年份都不是闰年。
要实现这个功能,你可以创建一个名为`days_in_month()`的函数,接受两个参数:年份(int)和月份(int),并根据给定规则返回相应月份的天数。下面是C语言的代码实现:
```c
#include <stdio.h>
// 判断是否为闰年
int is_leap_year(int year) {
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0)
return 1; // 是闰年
else
return 0; // 不是闰年
} else {
return 1; // 是闰年
}
} else {
return 0; // 不是闰年
}
}
// 根据年份和月份计算天数
int days_in_month(int year, int month) {
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31;
case 4:
case 6:
case 9:
case 11:
return 30;
case 2:
if (is_leap_year(year))
return 29;
else
return 28;
default:
printf("Invalid month number. Please enter a valid value between 1 and 12.\n");
return -1; // 表示输入错误
}
}
int main() {
int year, month;
printf("Enter the year: ");
scanf("%d", &year);
printf("Enter the month: ");
scanf("%d", &month);
if (month >= 1 && month <= 12) {
int day_count = days_in_month(year, month);
if (day_count != -1)
printf("The number of days in %d-%02d is %d\n", year, month, day_count);
else
printf("Day count not calculated due to invalid input.\n");
} else {
printf("Month should be between 1 and 12.\n");
}
return 0;
}
```
在这个程序中,首先定义了一个`is_leap_year()`函数来检查给定的年份是否是闰年。然后在`days_in_month()`函数中,我们使用switch语句根据不同月份返回相应的天数,特殊情况是2月,如果输入的是闰年则返回29天,否则返回28天。
在主函数`main()`中,用户会被要求输入年份和月份,程序会根据这些值调用`days_in_month()`函数并输出结果。如果输入的月份不在1到12之间,程序会给出提示。
阅读全文
相关推荐
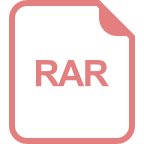
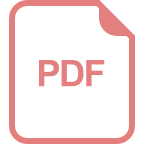
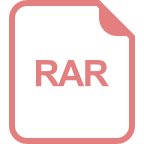
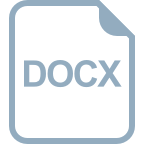
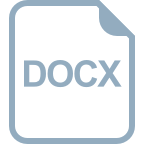
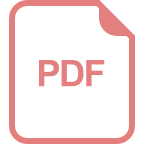
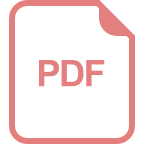
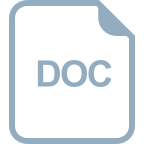
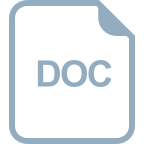





