selenium 图片验证码
时间: 2023-11-12 18:06:01 浏览: 236
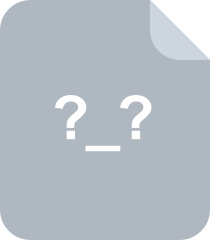
图片验证码
Selenium可以用于解决图片验证码的问题。一般来说,可以通过以下步骤来实现:
1. 使用Selenium打开网页并输入用户名和密码。
2. 使用Selenium获取验证码图片的URL,并下载图片到本地。
3. 使用Python的第三方库(如Pillow)读取图片,并对图片进行处理,以便于后续的识别。
4. 使用Python的第三方库(如Tesseract)对图片进行OCR识别,得到验证码的文本。
5. 使用Selenium输入验证码并提交表单。
下面是一个示例代码,用于解决滑块验证码的问题:
```python
from selenium import webdriver
from PIL import Image
import time
# 设置Chrome驱动路径
chrome_path = r"C:\Users\11248\AppData\Local\Google\Chrome\Application\chromedriver.exe"
# 打开网页
url = 'https://www.xxx.com/login'
driver = webdriver.Chrome(chrome_path)
driver.get(url)
# 输入用户名和密码
username = driver.find_element_by_id('username')
password = driver.find_element_by_id('password')
username.send_keys('your_username')
password.send_keys('your_password')
# 获取验证码图片的URL,并下载图片到本地
img_url = driver.find_element_by_id('captcha').get_attribute('src')
driver.get_screenshot_as_file('screenshot.png')
img = Image.open('screenshot.png')
left = driver.find_element_by_id('captcha').location['x']
top = driver.find_element_by_id('captcha').location['y']
right = left + driver.find_element_by_id('captcha').size['width']
bottom = top + driver.find_element_by_id('captcha').size['height']
img.crop((left, top, right, bottom)).save('captcha.png')
# 对图片进行处理,以便于后续的识别
captcha = Image.open('captcha.png')
captcha = captcha.convert('L')
captcha = captcha.point(lambda x: 0 if x < 150 else 255)
# 对图片进行OCR识别,得到验证码的文本
text = pytesseract.image_to_string(captcha, lang='eng', config='--psm 7')
print('验证码为:', text)
# 输入验证码并提交表单
captcha_input = driver.find_element_by_id('captcha_input')
captcha_input.send_keys(text)
submit_button = driver.find_element_by_id('submit_button')
submit_button.click()
```
阅读全文
相关推荐
















