编程实现:分别采用结构体数组和链表存储学生信息(包括学号、姓名、各科成绩),按成绩(总分)排序,按学号、姓名查找。
时间: 2023-12-23 14:04:04 浏览: 139
好的,我可以为您提供这样的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
typedef struct Student {
char id[20]; // 学号
char name[20]; // 姓名
int score[3]; // 三门科目成绩
int total; // 总分
} Student;
// 定义链表结构体
typedef struct Node {
Student data; // 数据域
struct Node *next; // 指针域
} Node;
// 结构体数组排序函数
void sort(Student *stu, int len) {
int i, j, k;
Student tmp;
for (i = 0; i < len - 1; i++) {
k = i;
for (j = i + 1; j < len; j++) {
if (stu[j].total > stu[k].total) {
k = j;
}
}
if (k != i) {
tmp = stu[i];
stu[i] = stu[k];
stu[k] = tmp;
}
}
}
// 链表排序函数
void sort_list(Node *head) {
Node *p, *q;
Student tmp;
for (p = head; p != NULL; p = p->next) {
for (q = p->next; q != NULL; q = q->next) {
if (q->data.total > p->data.total) {
tmp = p->data;
p->data = q->data;
q->data = tmp;
}
}
}
}
// 结构体数组查找函数
void search(Student *stu, int len, char *key, int type) {
int i;
for (i = 0; i < len; i++) {
if (type == 0 && strcmp(stu[i].id, key) == 0) {
printf("ID: %s, Name: %s, Score: %d %d %d, Total: %d\n", stu[i].id, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].total);
return;
} else if (type == 1 && strcmp(stu[i].name, key) == 0) {
printf("ID: %s, Name: %s, Score: %d %d %d, Total: %d\n", stu[i].id, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].total);
return;
}
}
printf("Not found.\n");
}
// 链表查找函数
void search_list(Node *head, char *key, int type) {
Node *p;
for (p = head; p != NULL; p = p->next) {
if (type == 0 && strcmp(p->data.id, key) == 0) {
printf("ID: %s, Name: %s, Score: %d %d %d, Total: %d\n", p->data.id, p->data.name, p->data.score[0], p->data.score[1], p->data.score[2], p->data.total);
return;
} else if (type == 1 && strcmp(p->data.name, key) == 0) {
printf("ID: %s, Name: %s, Score: %d %d %d, Total: %d\n", p->data.id, p->data.name, p->data.score[0], p->data.score[1], p->data.score[2], p->data.total);
return;
}
}
printf("Not found.\n");
}
int main() {
int len, i;
printf("Please input the number of students: ");
scanf("%d", &len);
// 结构体数组存储
Student *stu = (Student *)malloc(len * sizeof(Student));
for (i = 0; i < len; i++) {
printf("Please input the information of student %d:\n", i+1);
printf("ID: ");
scanf("%s", stu[i].id);
printf("Name: ");
scanf("%s", stu[i].name);
printf("Score 1: ");
scanf("%d", &stu[i].score[0]);
printf("Score 2: ");
scanf("%d", &stu[i].score[1]);
printf("Score 3: ");
scanf("%d", &stu[i].score[2]);
stu[i].total = stu[i].score[0] + stu[i].score[1] + stu[i].score[2];
}
sort(stu, len);
printf("The result sorted by total score:\n");
for (i = 0; i < len; i++) {
printf("ID: %s, Name: %s, Score: %d %d %d, Total: %d\n", stu[i].id, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2], stu[i].total);
}
char key[20];
printf("Please input the ID or name you want to search: ");
scanf("%s", key);
printf("Search result:\n");
search(stu, len, key, 0);
search(stu, len, key, 1);
// 链表存储
Node *head = NULL, *p, *q;
for (i = 0; i < len; i++) {
p = (Node *)malloc(sizeof(Node));
p->data = stu[i];
p->next = NULL;
if (head == NULL) {
head = p;
} else {
q->next = p;
}
q = p;
}
sort_list(head);
printf("The result sorted by total score:\n");
for (p = head; p != NULL; p = p->next) {
printf("ID: %s, Name: %s, Score: %d %d %d, Total: %d\n", p->data.id, p->data.name, p->data.score[0], p->data.score[1], p->data.score[2], p->data.total);
}
printf("Please input the ID or name you want to search: ");
scanf("%s", key);
printf("Search result:\n");
search_list(head, key, 0);
search_list(head, key, 1);
return 0;
}
```
此代码中,我们定义了一个 `Student` 结构体来存储学生信息,包括学号、姓名和三门科目成绩,以及一个 `total` 字段来记录总分。然后,我们又定义了一个 `Node` 结构体作为链表节点,其中 `data` 字段是一个 `Student` 结构体,`next` 字段是一个指向下一个节点的指针。接着,我们分别实现了结构体数组和链表排序函数、查找函数。最后,在 `main` 函数中,我们先用结构体数组存储学生信息,并进行排序和查找;然后,我们用链表存储同样的学生信息,并进行排序和查找。
阅读全文
相关推荐
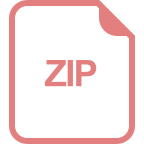
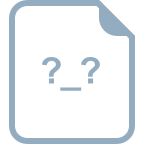

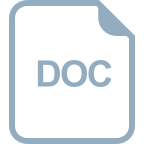
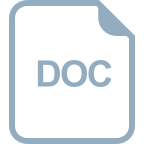
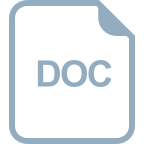




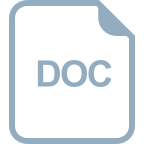
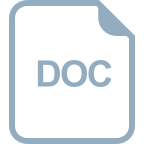
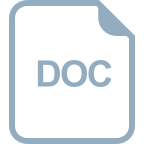




