geoserver发布geojson格式的shp文件的java代码
时间: 2023-10-15 15:06:03 浏览: 164
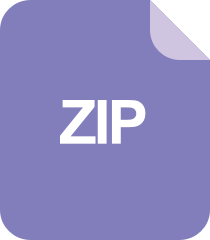
java使用代码上传tif至geoserver发布服务并设置坐标系和style样式.zip
你可以使用 GeoTools 库来实现将 SHP 文件发布为 GeoJSON 格式的服务。下面是一个示例代码:
```java
import org.geoserver.catalog.Catalog;
import org.geoserver.catalog.CoverageStoreInfo;
import org.geoserver.catalog.DataStoreInfo;
import org.geoserver.catalog.FeatureTypeInfo;
import org.geoserver.catalog.NamespaceInfo;
import org.geoserver.catalog.StoreInfo;
import org.geoserver.catalog.WorkspaceInfo;
import org.geoserver.catalog.impl.CatalogImpl;
import org.geoserver.data.util.IOUtils;
import org.geoserver.platform.GeoServerExtensions;
import org.geoserver.wfs.WFSServiceImpl;
import org.geotools.data.DataStore;
import org.geotools.data.DataStoreFinder;
import org.geotools.data.FeatureSource;
import org.geotools.data.FeatureStore;
import org.geotools.data.Transaction;
import org.geotools.data.simple.SimpleFeatureCollection;
import org.geotools.data.simple.SimpleFeatureSource;
import org.geotools.data.simple.SimpleFeatureStore;
import org.geotools.feature.FeatureCollection;
import org.geotools.geojson.feature.FeatureJSON;
import org.geotools.geometry.jts.ReferencedEnvelope;
import org.json.simple.JSONObject;
import org.json.simple.parser.JSONParser;
import org.opengis.feature.Property;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import org.opengis.filter.Filter;
import org.opengis.referencing.crs.CoordinateReferenceSystem;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletRequest;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
@RestController
@RequestMapping("/geojson")
public class GeoJSONController {
private Catalog catalog;
public GeoJSONController() {
this.catalog = new CatalogImpl();
}
@RequestMapping(value = "/{workspace}/{datastore}/{layer}", method = RequestMethod.GET, produces = MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<String> getGeoJSON(@PathVariable("workspace") String workspaceName,
@PathVariable("datastore") String datastoreName,
@PathVariable("layer") String layerName,
HttpServletRequest request) {
try {
WorkspaceInfo workspace = catalog.getWorkspaceByName(workspaceName);
DataStoreInfo dataStore = catalog.getDataStoreByName(datastoreName, workspace);
FeatureTypeInfo featureType = catalog.getFeatureTypeByName(workspaceName, layerName);
DataStore datastore = DataStoreFinder.getDataStore(dataStore.getConnectionParameters());
SimpleFeatureSource featureSource = datastore.getFeatureSource(featureType.getName());
FeatureCollection<SimpleFeatureType, SimpleFeature> collection = featureSource.getFeatures();
SimpleFeatureCollection features = (SimpleFeatureCollection) collection;
// Convert SimpleFeatureCollection to GeoJSON
FeatureJSON featureJSON = new FeatureJSON();
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
featureJSON.writeFeatureCollection(features, outputStream);
return ResponseEntity.ok(outputStream.toString());
} catch (Exception e) {
return ResponseEntity.status(500).body("Error: " + e.getMessage());
}
}
}
```
在此代码中,我们首先获取了指定的工作空间、数据存储和要素类型。然后,我们使用 `DataStoreFinder` 类创建一个 `DataStore` 实例,并从中获取要素源。接下来,我们使用 GeoTools 库的 `FeatureJSON` 类将 `SimpleFeatureCollection` 转换为 GeoJSON 字符串,并返回该字符串作为响应。
阅读全文
相关推荐
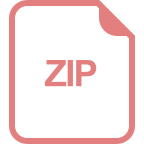
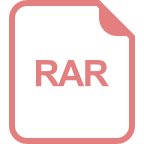















