数据结构活期储蓄账目管理系统
时间: 2023-12-22 11:04:26 浏览: 110
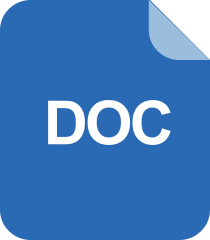
活期储蓄账目管理 数据结构
活期储蓄账目管理系统可以使用链表数据结构来实现。其中,横向链表用于存储每个用户的存取款记录等信息,纵向链表用于存储用户的账号ID等信息。
以下是一个简单的示例代码,演示了如何使用链表数据结构实现活期储蓄账目管理系统:
```python
class Account:
def __init__(self, account_id):
self.account_id = account_id
self.transactions = []
class Transaction:
def __init__(self, transaction_type, amount):
self.transaction_type = transaction_type
self.amount = amount
class AccountManagementSystem:
def __init__(self):
self.accounts = []
def insert_account(self, account_id):
account = Account(account_id)
self.accounts.append(account)
def delete_account(self, account_id):
for account in self.accounts:
if account.account_id == account_id:
self.accounts.remove(account)
break
def deposit(self, account_id, amount):
for account in self.accounts:
if account.account_id == account_id:
transaction = Transaction("Deposit", amount)
account.transactions.append(transaction)
break
def withdraw(self, account_id, amount):
for account in self.accounts:
if account.account_id == account_id:
transaction = Transaction("Withdraw", amount)
account.transactions.append(transaction)
break
def find_account(self, account_id):
for account in self.accounts:
if account.account_id == account_id:
return account
def display_transactions(self, account_id):
account = self.find_account(account_id)
if account:
for transaction in account.transactions:
print(f"Transaction Type: {transaction.transaction_type}, Amount: {transaction.amount}")
else:
print("Account not found.")
# 创建账目管理系统对象
ams = AccountManagementSystem()
# 插入账户
ams.insert_account("A001")
ams.insert_account("A002")
# 存款
ams.deposit("A001", 1000)
ams.deposit("A002", 2000)
# 取款
ams.withdraw("A001", 500)
ams.withdraw("A002", 1000)
# 显示账户的交易记录
ams.display_transactions("A001")
ams.display_transactions("A002")
```
这段代码演示了一个简单的活期储蓄账目管理系统,其中包括插入账户、删除账户、存款、取款和显示交易记录等功能。你可以根据实际需求进行扩展和修改。
阅读全文
相关推荐
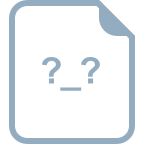
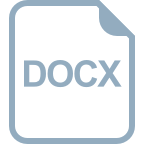
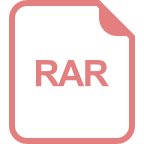
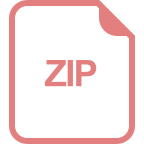
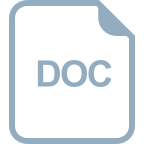
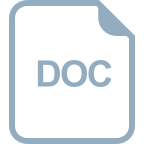



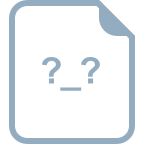
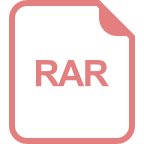
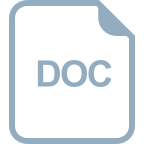