你这里面怎么修改路径啊mounted() { const fileInput = document.createElement('input'); fileInput.type = 'file'; fileInput.multiple = true; fileInput.accept = 'video/mp4'; // 允许选择MP4格式的视频文件。这是必需的,因为Web Worker和Chrome only。'; fileInput.addEventListener('change', event => { const files = event.target.files; const fileURLs = []; // 遍历文件列表,获取文件 URL for (let i = 0; i < files.length; i++) { const file = files[i]; const fileURL = URL.createObjectURL(file); fileURLs.push(fileURL); } // 将文件 URL 存入 videoList 数组中 this.videoList = fileURLs; });
时间: 2024-04-03 12:35:40 浏览: 16
这段代码是在Vue的mounted钩子函数中创建了一个input元素,用于选择视频文件,并将选择的文件URL存储在videoList数组中。如果你想修改路径,可以通过修改accept属性来限定选择的文件类型,或者在遍历文件列表时修改获取文件URL的逻辑来改变路径。例如,如果你想允许选择所有类型的文件,可以将accept属性设置为空字符串。如果你想从不同的文件路径中选择文件,可以在创建input元素时设置directory属性,并将其值设置为true,这样就可以选择文件夹而不是文件了。
相关问题
const script = document.createElement('script'); 在vue里面怎么写
在 Vue 中,可以通过使用 `this.$el.appendChild(script)` 来向当前组件的 DOM 元素中添加新的 `<script>` 标签。所以你可以这样写:
```
const script = document.createElement('script');
this.$el.appendChild(script);
```
需要注意的是,如果你需要在 Vue 中使用动态添加的 `<script>` 标签,最好将该标签添加到 `mounted()` 钩子函数中。这可以确保在组件挂载完成后,才会开始加载该标签所引用的脚本文件。
将以下代码转换为vue3的代码:const username = document.getElementById('username')
在Vue 3中,可以使用`ref`函数来创建响应式变量。所以可以将上述的代码转换为:
```
import { ref } from 'vue'
const username = ref(null)
// 在mounted钩子中获取DOM元素
mounted() {
username.value = document.getElementById('username')
}
```
在模板中,可以这样使用:
```
<template>
<div>
<input type="text" :value="username.value" />
</div>
</template>
```
需要注意的是,在Vue 3中,不再需要使用`$refs`来获取DOM元素的引用。
相关推荐
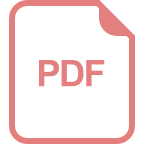
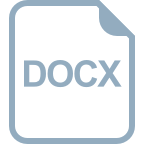
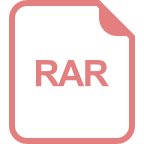













