利用双向链表实现通讯录的建立、删除、检索和列表,并可保存至文件,可重新装入。 c语言代码实现
时间: 2024-11-04 11:15:23 浏览: 7
在C语言中,我们可以利用双向链表数据结构来实现一个简单的通讯录功能。这里是一个基本的示例,展示了如何创建、删除、查找和保存通讯录到文件的逻辑:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构体
typedef struct Node {
char name[50];
char phone[20];
struct Node* prev;
struct Node* next;
} Contact;
// 创建新节点
Contact* createNode(char* name, char* phone) {
Contact* newNode = (Contact*)malloc(sizeof(Contact));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
strcpy(newNode->name, name);
strcpy(newNode->phone, phone);
newNode->prev = NULL;
newNode->next = NULL;
return newNode;
}
// 添加联系人到链表
void addContact(Contact** head, Contact* node) {
if (*head == NULL) {
*head = node;
} else {
node->prev = *head;
(*head)->next = node;
*head = node;
}
}
// 删除联系人
void deleteContact(Contact** head, char* name) {
Contact* current = *head;
while (current != NULL && strcmp(current->name, name) != 0) {
current = current->next;
}
if (current != NULL) {
if (current->prev != NULL)
current->prev->next = current->next;
else
*head = current->next;
if (current->next != NULL)
current->next->prev = current->prev;
free(current);
}
}
// 搜索并打印联系人
void searchContact(Contact* head, char* name) {
Contact* current = head;
while (current != NULL) {
if (strcmp(current->name, name) == 0) {
printf("Name: %s, Phone: %s\n", current->name, current->phone);
break;
}
current = current->next;
}
}
// 保存通讯录到文件
void saveToFile(const char* filename, Contact* head) {
FILE* file = fopen(filename, "w");
if (file == NULL) {
printf("Failed to open file.\n");
return;
}
Contact* current = head;
while (current != NULL) {
fprintf(file, "%s, %s\n", current->name, current->phone);
current = current->next;
}
fclose(file);
printf("Contacts saved to file.\n");
}
// 从文件读取并重建通讯录
Contact* loadFromFile(const char* filename) {
Contact* head = NULL;
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("Failed to open file.\n");
return head;
}
char line[100], name[50], phone[20];
while (fgets(line, sizeof(line), file)) {
if (sscanf(line, "%s, %s", name, phone) == 2) {
Contact* newNode = createNode(name, phone);
if (newNode != NULL) {
addContact(&head, newNode);
}
}
}
fclose(file);
return head;
}
int main() {
// 初始化链表
Contact* contactList = NULL;
// 添加联系人
Contact* john = createNode("John Doe", "1234567890");
addContact(&contactList, john);
// 删除联系人
deleteContact(&contactList, "John Doe");
// 搜索并打印联系人
searchContact(contactList, "Jane Smith"); // 示例搜索
// 保存到文件
saveToFile("contacts.txt", contactList);
// 重新加载通讯录
Contact* loadedList = loadFromFile("contacts.txt");
// 结束前释放内存
while (loadedList != NULL) {
Contact* temp = loadedList;
loadedList = loadedList->next;
free(temp);
}
return 0;
}
```
请注意,这个示例仅作为一个基础教学用例,实际应用中可能需要处理更多的错误情况和边界条件。同时,由于C标准库对文件操作的限制,建议在生产环境中使用更可靠的文件操作函数和错误处理机制。
阅读全文
相关推荐
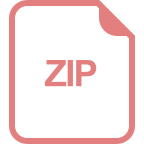
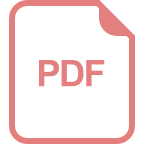
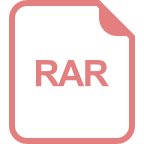
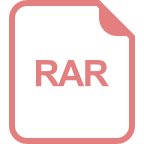
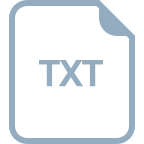
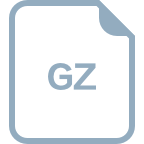
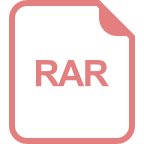
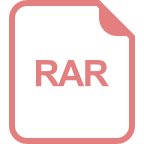
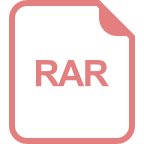
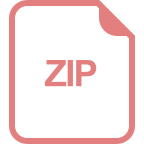
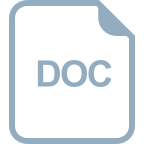
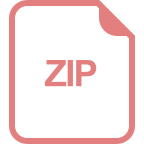