C语言实现单链表的产生,查找,插入,删除,输出
时间: 2023-10-22 12:15:56 浏览: 41
```c
#include <stdio.h>
#include <stdlib.h>
// 定义结构体
typedef struct Node {
int data;
struct Node *next;
} Node;
// 创建链表
Node *createList() {
Node *head = NULL, *tail = NULL, *p = NULL;
int x;
printf("请输入链表中的元素,以-1结束:");
while (1) {
scanf("%d", &x);
if (x == -1) {
break;
}
p = (Node *)malloc(sizeof(Node));
p->data = x;
p->next = NULL;
if (head == NULL) {
head = tail = p;
} else {
tail->next = p;
tail = p;
}
}
return head;
}
// 查找元素
int searchList(Node *head, int x) {
int index = 0;
Node *p = head;
while (p != NULL) {
if (p->data == x) {
return index;
}
p = p->next;
index++;
}
return -1;
}
// 插入元素
Node *insertList(Node *head, int x, int index) {
Node *p = head, *q = NULL;
int i = 0;
while (p != NULL && i < index) {
q = p;
p = p->next;
i++;
}
if (i != index) {
printf("插入位置不合法!\n");
return head;
}
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = x;
if (q == NULL) {
newNode->next = head;
head = newNode;
} else {
newNode->next = p;
q->next = newNode;
}
return head;
}
// 删除元素
Node *deleteList(Node *head, int index) {
Node *p = head, *q = NULL;
int i = 0;
while (p != NULL && i < index) {
q = p;
p = p->next;
i++;
}
if (i != index) {
printf("删除位置不合法!\n");
return head;
}
if (q == NULL) {
head = p->next;
} else {
q->next = p->next;
}
free(p);
return head;
}
// 输出链表
void printList(Node *head) {
Node *p = head;
printf("链表中的元素为:");
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
Node *head = createList();
printList(head);
int x, index;
printf("请输入要查找的元素:");
scanf("%d", &x);
index = searchList(head, x);
if (index == -1) {
printf("不存在该元素!\n");
} else {
printf("该元素在链表中的位置为:%d\n", index);
}
printf("请输入要插入的元素和插入位置:");
scanf("%d %d", &x, &index);
head = insertList(head, x, index);
printList(head);
printf("请输入要删除的元素位置:");
scanf("%d", &index);
head = deleteList(head, index);
printList(head);
return 0;
}
```
相关推荐
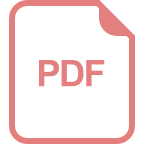
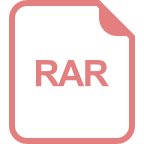
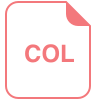
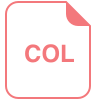
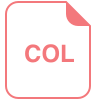
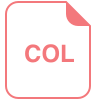
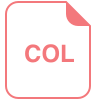









