java代码使用多线程发送5000ciwebsocket请求
时间: 2023-11-09 12:04:18 浏览: 43
以下是使用Java多线程发送5000次WebSocket请求的示例代码:
```java
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import org.java_websocket.client.WebSocketClient;
import org.java_websocket.handshake.ServerHandshake;
public class TestWebSocketClient {
private static final int THREAD_COUNT = 50;
private static final int MESSAGE_COUNT = 100;
public static void main(String[] args) throws URISyntaxException, InterruptedException {
final URI uri = new URI("ws://localhost:8080/websocket");
final ExecutorService executorService = Executors.newFixedThreadPool(THREAD_COUNT);
final CountDownLatch latch = new CountDownLatch(THREAD_COUNT);
for (int i = 0; i < THREAD_COUNT; i++) {
executorService.execute(new Runnable() {
@Override
public void run() {
try {
WebSocketClient client = new WebSocketClient(uri) {
@Override
public void onOpen(ServerHandshake handshake) {
System.out.println("Connected");
}
@Override
public void onMessage(String message) {
System.out.println("Received message: " + message);
}
@Override
public void onClose(int code, String reason, boolean remote) {
System.out.println("Disconnected");
}
@Override
public void onError(Exception ex) {
ex.printStackTrace();
}
};
client.connectBlocking();
for (int i = 0; i < MESSAGE_COUNT; i++) {
client.send("Hello, world!");
}
client.closeBlocking();
} catch (InterruptedException | URISyntaxException | IOException e) {
e.printStackTrace();
} finally {
latch.countDown();
}
}
});
}
latch.await();
executorService.shutdown();
}
}
```
这个示例代码使用了Java-WebSocket库(org.java_websocket),通过创建多个WebSocket客户端,每个客户端发送多个消息,来模拟发送5000次WebSocket请求的场景。其中,THREAD_COUNT和MESSAGE_COUNT可以根据实际需求进行修改。
相关推荐
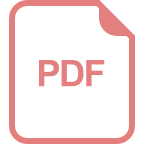














